Standard Template Library (STL) in C++
The Standard Template Library (STL) in C++ is a powerful collection of template classes to implement common data structures and algorithms. It includes containers (like vectors, lists, and maps), iterators to navigate through these containers, and algorithms to manipulate data. Mastering STL can significantly improve the performance and efficiency of your C++ programs.
Lets Go!
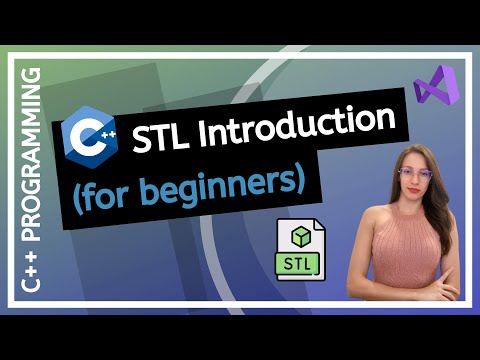
Standard Template Library (STL) in C++
Lesson 10
Learn to effectively use containers, iterators, algorithms, and functors in C++ to build efficient and optimized applications. Understand how to leverage STL's flexibility in solving various programming challenges.
Get Started 🍁Introduction to C++ Standard Template Library (STL)
Welcome to our new course where we dive into the world of C++ standard template library, commonly known as STL. In this introductory video, we will explore what STL is, why it is essential, and take a closer look at its key components.
Have you ever wondered what makes C++ programming more efficient and powerful? Join us on this journey to uncover the magic of STL and unleash its full potential.
Course Overview:
- What is STL?: A toolbox of tools for building C++ programs
- Containers: Storage units for data processing
- Algorithms: Instructions for data manipulation
- Function Objects: Customize your algorithms
- Iterators: Navigate through data efficiently
Why STL?
STL is highly efficient due to its reliance on templates, allowing generic code to work with various data types seamlessly. Embracing STL can streamline your code, optimize performance, reduce bugs, and enhance scalability.
Practical Programming Course:
For those eager to put theory into practice, consider joining our practical programming course. Together, we will build a comprehensive application, covering beginner to advanced programming concepts like object-oriented programming, data structures, algorithms, and more.
Challenge yourself to solve real-world problems and acquire transferable skills applicable across multiple programming languages. Don't miss this opportunity to level up your programming expertise!
Ready to embark on your coding journey? Join our Practical Programming Course and unlock your full potential in the world of software development.
Thank you for watching, and we look forward to guiding you through the exciting realm of C++ and STL. See you in the next video! 🚀
Main Concepts of C++ Standard Template Library
-
STL Overview: The STL (Standard Template Library) is a library in C++ that serves as a toolbox containing various tools for building programs.
-
Containers: Containers in STL are used to store data that requires processing, such as vectors, lists, and maps. Each type of container has different purposes and capabilities.
-
Algorithms: Algorithms in STL are sets of instructions for processing data, such as sorting, comparing, and searching. STL provides predefined algorithms, but custom algorithms can also be created using function objects (functors).
-
Function Objects (Functors): Function objects allow for the creation of custom algorithms or customization of existing algorithms in STL, providing flexibility in data processing.
-
Iterators: Iterators in STL are used to navigate through data within containers, similar to pointers in programming. They help in accessing and modifying elements in containers efficiently.
-
Efficiency: STL is efficient due to its reliance on templates, allowing for generic code that can work with different data types. Templates reduce code redundancy, optimize code maintenance, and enhance performance.
By understanding and utilizing these main concepts of the C++ Standard Template Library, programmers can write clean, efficient, and scalable code for various applications.
Practical Applications of C++ Standard Template Library (STL)
Let's dive into some hands-on practical applications of the concepts discussed in the video:
Step 1: Implementing Containers
-
Declare a vector container to store integer values.
std::vector<int> myVector;
-
Add some elements to the vector.
myVector.push_back(10); myVector.push_back(20);
Step 2: Applying Algorithms
-
Use the
std::sort
algorithm to sort the elements in the vector.std::sort(myVector.begin(), myVector.end());
-
Utilize other algorithms like
std::find
,std::count
, etc., to manipulate the data in the container.
Step 3: Customizing Algorithms with Functors
-
Define a functor to create a custom sorting order for students.
struct StudentSortFunctor { bool operator()(const Student& s1, const Student& s2) const { return s1.getGrade() < s2.getGrade(); } };
-
Apply the custom functor to your sorting algorithm for students.
Step 4: Navigating Data with Iterators
-
Use iterators to loop through the elements in your container.
for (auto it = myVector.begin(); it != myVector.end(); ++it) { std::cout << *it << " "; }
-
Experiment with different types of iterators like
std::vector::iterator
,std::vector::const_iterator
, etc.
Now, it's your turn to experiment with these practical applications of C++ STL! Feel free to modify the code snippets above and try out different scenarios to deepen your understanding of STL concepts. Happy coding!
Test your Knowledge
Which STL container is best for storing unique elements in sorted order?
Which STL container is best for storing unique elements in sorted order?
Advanced Insights into C++ Standard Template Library
In addition to the foundational knowledge shared in the introductory video, delving deeper into the C++ Standard Template Library (STL) can provide a profound understanding of its components and functionalities. Let's explore some advanced insights into STL:
Templates for Efficient Generic Code
One of the key features that make STL highly efficient is its reliance on templates. Templates allow developers to work with various data types using the same code structure. This means that instead of creating multiple functions for different data types, you can write a single function that can handle multiple data types. This leads to reduced code complexity, easier maintenance, enhanced readability, fewer bugs, and scalability. Understanding and leveraging templates in STL can significantly streamline your programming tasks.
Curiosity Question: How can templates in STL improve code reusability and scalability in large software projects?
Custom Algorithms with Functors
While STL provides a vast array of predefined algorithms for data processing, there may be instances where you need to tailor an algorithm to specific requirements. This is where function objects, also known as functors, come into play. Functors enable you to write custom algorithms or customize existing ones to suit your unique needs. By utilizing functors in STL, you can enhance the flexibility and adaptability of your codebase.
Curiosity Question: What criteria should be considered when designing and implementing a custom algorithm using functors in STL?
Leveraging Iterators for Data Navigation
Iterators play a crucial role in traversing and manipulating data stored within containers in STL. Comparable to pointers in traditional programming, iterators act as navigational tools within containers, allowing you to access and process elements efficiently. Mastering the usage of iterators in STL empowers you to maneuver through data structures effectively, enhancing your overall programming proficiency.
Curiosity Question: How can iterators in STL optimize data traversal and manipulation compared to traditional loop structures?
By exploring these advanced insights into the C++ Standard Template Library, you can deepen your knowledge and proficiency in utilizing its powerful tools and functionalities. Stay tuned for upcoming videos where we will delve into practical examples and real-world applications of these advanced STL concepts. Happy coding!
For more in-depth learning about C++ programming and practical application in building software projects, consider joining our Practical Programming Course to acquire career-ready skills and problem-solving abilities across various programming languages. Join our exclusive developer community and sharpen your programming expertise today!
##Additional Resources for C++ Standard Template Library (STL)
- C++ Standard Library - Wikipedia
- A Tour of C++ Standard Library - The C++ Programming Language Book
- Bjarne Stroustrup's official website for C++
- STL Containers Cheat Sheet
- STL Algorithms Guide
- STL Functions and Iterators Tutorial - Tutorialspoint
Dive deeper into the world of C++ STL by exploring these resources. Enhance your understanding and mastery of containers, algorithms, function objects, iterators, and templates. Happy learning! 🚀
Practice
Task
Task: Implement a program that uses map
to count word frequency in a sentence.
Task: Create a program that sorts a set
of integers in descending order.
Task: Write a program that removes duplicate elements from a vector using unique()
and erase()
.
Task: Use a queue
to simulate a waiting line system where people are added and removed in FIFO order.
Task: Implement a function that finds the largest element in a vector
using max_element()
.
Task: Create a program that reads a file and stores the words in a set
to ensure uniqueness.
Task: Implement a program that uses a deque
for efficient push and pop from both ends.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Learn Ruby Programming Online with Step-by-Step Video Tutorials
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Learn Java Programming Online with Step-by-Step Video Tutorials
Explore our Java Programming course online with engaging video tutorials and practical guides to boost your skills and knowledge.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Python Programming
Learn how to use Python for general programming, automation, and problem-solving.