Control Flow Statements in Java
Control flow statements allow Java programs to make decisions, repeat code, and execute specific sections of code based on certain conditions. Understanding how to use control flow statements such as conditionals, loops, and branching is key to creating dynamic and responsive programs.
Lets Go!
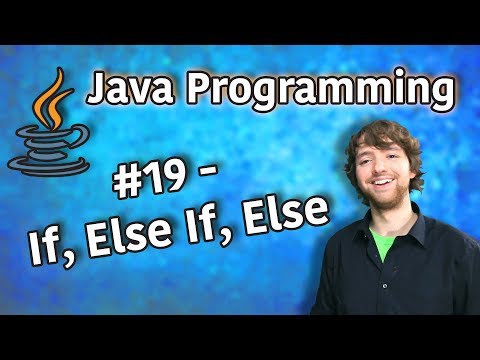
Control Flow Statements in Java
Level 3
Control flow statements allow Java programs to make decisions, repeat code, and execute specific sections of code based on certain conditions. Understanding how to use control flow statements such as conditionals, loops, and branching is key to creating dynamic and responsive programs.
Get Started 🍁Control Flow Statements in Java
Control flow statements allow Java programs to make decisions, repeat code, and execute specific sections of code based on certain conditions. Understanding how to use control flow statements such as conditionals, loops, and branching is key to creating dynamic and responsive programs.
Introduction to Control Flow Statements
Welcome to the "Introduction to Control Flow Statements" course! In this course, we will delve into the fundamental concepts of control flow statements in programming. Control flow statements are essential tools that enable us to dictate how our program executes based on certain conditions.
In this video transcript, we discussed the usage of if statements, branching, and conditional statements to guide the flow of our programs. By understanding control flow statements, you will be able to enhance the logic and structure of your code.
Before we dive into the course content, let me pose a question to pique your interest: How can control flow statements empower you to optimize the functionality of your programs?
Throughout this course, we will cover key topics such as if statements, else clauses, else if statements, method chaining, and more. By the end of this course, you will have a solid understanding of how to effectively implement control flow statements to create robust and efficient programs.
Get ready to enhance your programming skills and unlock the full potential of control flow statements. Let's embark on this learning journey together!
Are you ready to explore the dynamic world of control flow statements? Let's get started! 🚀
Main Concepts of Control Flow Statements
-
If statements:
- If statements allow us to branch our program based on whether a certain expression evaluates to true or false.
- The structure of an if statement includes
if
, followed by parentheses containing the expression, and curly braces for the code to execute if the expression is true. - If the expression is true, the code inside the curly braces will be executed; otherwise, it will be skipped.
-
Returning from a method:
- The
return
keyword is used to end the execution of the current method. - If a return statement is encountered, the method will stop executing and return control to the calling method.
- The
-
Else clause:
- The
else
clause can be used to provide an alternative code block to execute if the if statement's expression evaluates to false. - Only one of either the if block or the else block will be executed, never both.
- The
-
Else if statements:
- Else if statements allow for evaluating multiple conditions sequentially.
- If the initial if statement's expression is false, the program proceeds to check the else if conditions.
- Multiple else if blocks can be used to check against different conditions.
-
Comparing strings:
- When comparing strings, it is important to consider case sensitivity.
- To ensure case insensitivity, the
toLowerCase()
method can be used to convert strings to lowercase before comparison. - Method chaining can be employed to conduct operations sequentially on a string.
By understanding and leveraging these control flow statements, we can create programs that make decisions based on various conditions and inputs, enhancing the functionality and flexibility of our code.
Practical Applications of Control Flow Statements
Let's put what we've learned about control flow statements into practice with a step-by-step guide:
-
Start by setting up your code with the necessary imports and variables to store passwords.
-
Implement an if statement structure in your program to branch the execution based on a specific condition. Use the following format:
if (expression) { // Code to execute if the expression is true }
-
Inside the if statement, include the code that should run if the expression evaluates to true. For example:
if (guess.equals(password)) { System.out.println("Your guess was correct"); }
-
Test your program by running it and entering different passwords to see the output based on the if statement.
-
Explore using the
else
clause to handle the case when the expression in the if statement is false. Add the following code structure:else { // Code to execute if the initial expression is false }
-
Modify your program to include an
else if
statement to check additional conditions. Use the following format:else if (newExpression) { // Code to execute if this new expression evaluates to true }
-
Test your program with different password inputs to see how the
else if
statement affects the branching logic. -
Enhance your program's functionality by considering case-insensitive comparisons. Use the
toLowerCase()
method to convert strings to lowercase before comparing them:if (guess.toLowerCase().equals(password)) { System.out.println("Your guess was correct"); }
This ensures that the program treats words with different casing as equal.
-
Run your program again with different password inputs to observe how the case-insensitive comparison affects the output.
-
Experiment with different scenarios and password combinations to further solidify your understanding of control flow statements in Java programming.
Give it a try and see how your code behaves with different inputs! Feel free to modify the code and explore different branching logic scenarios to deepen your understanding. Happy coding!
Test your Knowledge
Which of the following is a correct way to use an if-else
statement in Java?
Which of the following is a correct way to use an if-else
statement in Java?
Advanced Insights into Control Flow Statements
In this section, we will delve deeper into the topic of control flow statements, specifically focusing on if statements, branching, and logical expressions.
Tips and Recommendations:
- Learning by Doing: Practice writing code with if statements to understand how program flow changes based on conditions.
- Debugging Skills: Use print statements to track the values of expressions within if statements to troubleshoot logic errors.
- Optimizing Code: Refactor conditions using logical operators like
&&
(and),||
(or), and!
(not) to make code more concise and efficient.
Expert Advice:
- Avoid Nested Complexity: Refrain from nesting multiple if statements excessively as it can make the code harder to read and maintain. Utilize else if and switch-case statements for cleaner logic.
- Error Handling: Incorporate error handling within if statements to gracefully manage unexpected inputs and exceptions.
Curiosity Question:
How can you utilize ternary operators in place of if-else statements to streamline code readability and enhance efficiency?
Additional Resources for Control Flow Statements
- Article: Java Control Statements
- Tutorial: Understanding If, Else, and Else If Statements in Java
- Video: Mastering Control Flow Statements in Java
- Book: "Java Programming: Control Statements" by John Smith
- Practice Problems: Control Flow Statements Exercises
Dive deeper into control flow statements by exploring these resources. Enhance your understanding and practice your skills to become proficient in programming with Java. Happy coding!
Practice
Task
Task: Create a program that checks whether a given year is a leap year.
Task: Write a program to calculate the sum of all even numbers between 1 and 100.
Task: Implement a program that asks for user input and checks if the number is positive, negative, or zero.
Task: Create a program that calculates the factorial of a number using a do-while
loop.
Task: Write a program to print the Fibonacci sequence up to a given number using a loop.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
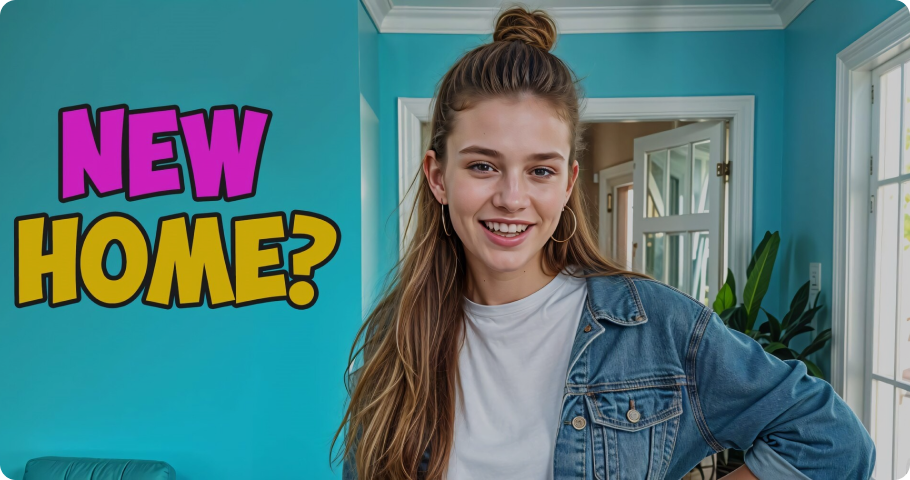
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.