Methods and Functions in Java
Methods in Java are blocks of code that perform a specific task, and functions are methods that can return a value. These building blocks allow for code reuse, modularity, and abstraction, making your programs more organized and efficient.
Lets Go!
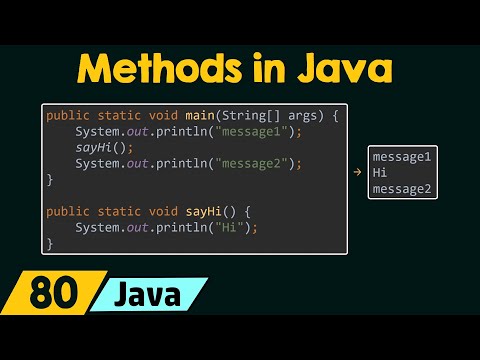
Methods and Functions in Java
Level 4
Methods in Java are blocks of code that perform a specific task, and functions are methods that can return a value. These building blocks allow for code reuse, modularity, and abstraction, making your programs more organized and efficient.
Get Started 🍁Methods and Functions in Java
Methods in Java are blocks of code that perform a specific task, and functions are methods that can return a value. These building blocks allow for code reuse, modularity, and abstraction, making your programs more organized and efficient.
Introduction to Methods in Java
Welcome to "Introduction to Methods in Java"! In this course, we will delve into the fundamental concepts of methods, an essential component of Java programming. Methods allow us to encapsulate code, promote reusability, and enhance the structure of our programs.
We will start by exploring void methods, which do not return a value. We will cover topics such as method declaration, parameter passing, and method invocation. Through practical examples and explanations, you will gain a strong understanding of how void methods operate within Java programs.
Next, we will move on to value-returning methods, which return a specific data type. You will learn about method signatures, return statements, and handling return values. By the end of this section, you will be able to create and call value-returning methods with confidence.
Additionally, we will discuss the importance of the return keyword and its role in controlling the flow of execution within methods. You will discover how to effectively use the return statement to exit methods and return values based on specific conditions.
Are you ready to unlock the power of methods in Java and elevate your programming skills? Let's embark on this learning journey together and explore the fascinating world of methods in Java! What mysteries and challenges do you think await us in this exploration? Join me as we unravel the secrets of Java methods and enhance our programming prowess. Let's dive in and start our adventure! 🚀🔍
Main Concepts of Methods in Java
-
Void Methods:
- A void method is a method that does not return a value. The return type of the method is void.
- Example:
public void sayHi() { System.out.println("Hi"); }
- In the method declaration, the keywords used include
public
,static
,void
, method name, and parameters (if any). The body of the method contains the code to be executed.
-
Value-Returning Methods:
- A value-returning method is a method that returns a value. The return type of the method is the type of data returned.
- Example:
public static int sum(int x, int y) { return x + y; }
- Parameters can be passed to the method, and the return keyword is used to return a value from the method.
-
Return Keyword:
- The
return
keyword is used to return a value from a method. - It can be used to exit a method early based on a condition.
- Example:
public void sayHi() { System.out.println("Hi"); return; }
- Incorrect use of the return keyword, such as returning a value that does not match the method's return type, can result in errors.
- The
By understanding void methods, value-returning methods, and the proper use of the return keyword, you can effectively work with methods in Java.
Practical Applications of Methods
Methods in Java offer a way to organize and reuse code efficiently. Let's dive into some practical applications based on the concepts discussed in the video.
Step-by-Step Guide:
-
Creating a Void Method:
- To create a void method, follow these steps:
public static void sayHi() { System.out.println("Hi"); }
- To create a void method, follow these steps:
-
Calling a Void Method:
- Call the
sayHi
method within themain
method:public static void main(String[] args) { System.out.println("Message 1"); sayHi(); System.out.println("Message 2"); }
- Call the
-
Creating a Value-Returning Method:
- Define a method that returns a value:
public static int sum(int X, int Y) { return X + Y; }
- Define a method that returns a value:
-
Calling a Value-Returning Method:
- Invoke the
sum
method with parameters:public static void main(String[] args) { System.out.println("Message 1"); int result = sum(1, 2); System.out.println(result); System.out.println("Message 2"); }
- Invoke the
Ready to Try It Out?
Go ahead and create a Java program using the provided steps. Experiment with void and value-returning methods to see how they work in action. Don't hesitate to tweak the code and explore different scenarios to deepen your understanding of Java methods. Your hands-on experience will solidify your grasp on these essential programming concepts. Have fun coding! 🚀
Test your Knowledge
What is the correct syntax for a method that takes two integers and returns their sum?
What is the correct syntax for a method that takes two integers and returns their sum?
Advanced Insights into Methods
In this section, we will delve deeper into the concept of methods, covering void methods, value-returning methods, the return keyword, and how to call methods effectively.
Void Methods
A void method, as discussed, does not return a value. The return type of a void method is "void." When creating a void method in Java, always remember to use the public
and static
keywords in the method signature. The header of the method contains modifiers, type, name, and parameters. Utilize the body of the method to write the code that will be executed when the method is called.
Tip: Use void methods when you need a block of code to perform actions without returning a specific result.
Curiosity question: How can you enhance the functionality of void methods by incorporating conditional statements?
Value-Returning Methods
Contrary to void methods, value-returning methods return a specific value. The return type of a value-returning method is the data type of the value being returned. Parameters can be passed to value-returning methods, allowing for more dynamic functionality in your code.
Recommendation: Utilize value-returning methods when you need to perform a calculation or generate a result to be used in other parts of your program.
Curiosity question: How can you modify a value-returning method to handle multiple return statements based on different conditions?
The Return Keyword
The return
keyword plays a crucial role in methods by explicitly returning a value or exiting the method. When using the return
keyword, ensure that the data being returned matches the method's return type. Misuse of the return
keyword can lead to errors and unreachable code segments.
Expert advice: Be mindful of the usage of the return
keyword to ensure a smooth flow of execution in your methods.
Curiosity question: Can you identify scenarios where strategically placing return
statements can optimize the performance of your methods?
By mastering the concepts of void methods, value-returning methods, and the return keyword, you can elevate your programming skills and build more efficient and structured Java programs. Stay tuned for further practice sessions to solidify your understanding of methods. Happy coding!
Additional Resources for Methods in Java
-
Java Methods Tutorial - A comprehensive tutorial on Java methods, including void methods and value-returning methods.
-
Oracle Java Documentation - Official documentation provided by Oracle on Java methods, covering topics such as method declaration and method calling.
-
GeeksforGeeks - Java Methods - An in-depth article on Java methods, explaining the concept of void methods, return types, and passing parameters.
-
Codecademy Java Course - An interactive Java course on Codecademy that covers methods, classes, and other essential Java programming concepts.
Explore these resources to deepen your understanding of methods in Java and enhance your programming skills! 🚀
Practice
Task
Task: Create a method that checks if a number is prime or not.
Task: Write a method that calculates the power of a number (e.g., x^y).
Task: Implement a program to reverse a string using a method.
Task: Create a method that sorts an array of integers in ascending order.
Task: Implement a method that finds the greatest common divisor (GCD) of two numbers.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
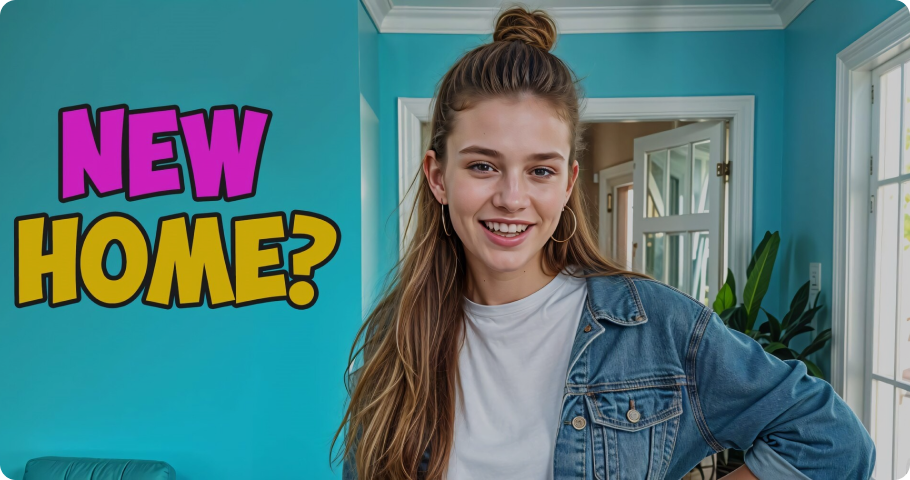
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.