Arrays and Strings in Java
Arrays in Java are used to store multiple values of the same type in a single variable, while Strings are used to represent sequences of characters. Mastering both arrays and strings is fundamental in handling collections of data and manipulating textual information in Java.
Lets Go!

Arrays and Strings in Java
Level 5
Arrays in Java are used to store multiple values of the same type in a single variable, while Strings are used to represent sequences of characters. Mastering both arrays and strings is fundamental in handling collections of data and manipulating textual information in Java.
Get Started 🍁Arrays and Strings in Java
Arrays in Java are used to store multiple values of the same type in a single variable, while Strings are used to represent sequences of characters. Mastering both arrays and strings is fundamental in handling collections of data and manipulating textual information in Java.
Introduction to [Course Topic]
Welcome to "Introduction to Arrays"!
In this course, we will dive deep into the fundamental concepts of arrays and their implementation in programming. Arrays are crucial data structures that allow us to store multiple values of the same data type in a single variable, providing us with efficiency and flexibility in handling data.
The ability to work with arrays is essential for any aspiring programmer, as arrays are used extensively in various applications and programming languages. Through this course, you will gain a solid understanding of how arrays work, how to create and manipulate arrays, and how to harness the power of arrays in your programs.
Before we begin, let me pose a question to spark your curiosity: How can a simple array help you manage and access multiple values efficiently in your code?
Whether you are new to programming or looking to enhance your skills, this course will equip you with the knowledge and skills needed to work with arrays effectively. Join us on this exciting journey to unlock the potential of arrays in programming!
Main Concepts of Arrays
-
Creating an Array:
- To create an array, you need to specify the type of elements it will hold (e.g., int) and provide a name for the array. For example,
int nums[] = {3, 7, 2, 4};
.
- To create an array, you need to specify the type of elements it will hold (e.g., int) and provide a name for the array. For example,
-
Accessing Elements in an Array:
- Each element in an array has an index number, starting from 0.
- To access a specific element in an array, you use the array name followed by the index number. For example,
nums[0]
will access the first element, which is 3.
-
Updating Elements in an Array:
- You can update the value of an element in an array by assigning a new value to that particular index. For example,
nums[1] = 6;
will change the second element to 6.
- You can update the value of an element in an array by assigning a new value to that particular index. For example,
-
Creating an Array Dynamically:
- If you want to create an array dynamically without specifying initial values, you can use the
new
keyword, likenew int[4];
. By default, all values will be set to 0.
- If you want to create an array dynamically without specifying initial values, you can use the
-
Using Loops with Arrays:
- Instead of accessing array elements individually, you can use loops to iterate through all elements in the array.
- A "for" loop is commonly used with arrays to iterate over each element efficiently, changing the index value accordingly.
-
Multi-Dimensional Arrays:
- In upcoming videos, you will learn about multi-dimensional arrays and how to manipulate them.
Understanding these main concepts will help you work effectively with arrays in programming, enabling you to store and manipulate multiple values efficiently.
Practical Applications of Arrays
Step-by-Step Guide:
-
Creating an Array:
- Declare an array with a specific type (e.g.,
int
) and name (e.g.,nums
). - Specify the values directly or dynamically.
int[] nums = {3, 7, 2, 4};
- Declare an array with a specific type (e.g.,
-
Accessing Array Elements:
- Use index numbers (starting from 0) to fetch values.
System.out.println(nums[0]); // Output: 3
- Use index numbers (starting from 0) to fetch values.
-
Updating Array Elements:
- Change a specific value by specifying the index.
nums[1] = 6; // Change the second value to 6 System.out.println(nums[1]); // Output: 6
- Change a specific value by specifying the index.
-
Creating Dynamic Arrays:
- Use the
new int[]
syntax to create an array of specified size.int[] nums = new int[4]; // Creates an array with four elements
- Use the
-
Iterating Through Array Elements:
- Use a
for
loop to print all values in the array.for (int i = 0; i < nums.length; i++) { System.out.println(nums[i]); }
- Use a
Try it Yourself:
- Create an array with your own set of values.
- Access a specific element and print it.
- Update an element in the array and verify the change.
- Experiment with creating dynamic arrays of different sizes.
- Use a loop to iterate through all elements and print them out.
Take these steps to practice working with arrays in Java and explore the possibilities they offer in programming tasks!
Test your Knowledge
How do you declare an array in Java?
How do you declare an array in Java?
Advanced Insights into Arrays
Now that we have covered the basics of arrays, let's delve into some advanced insights to enhance your understanding.
Accessing and Modifying Array Values
When working with arrays, it's crucial to understand how to access and modify values efficiently. Remember, array indexing starts at 0, not 1. This means the first element in an array will be at index 0, the second at index 1, and so on. By utilizing index numbers, you can easily fetch and update specific values within the array.
Tip: When updating array values, remember to adjust the index based on the zero-based indexing system.
Dynamic Array Initialization
Creating dynamic arrays allows flexibility in handling data. To initialize a dynamic array, use the syntax new int[size]
. By default, dynamic arrays are filled with zeros, which can be updated as needed. This approach offers versatility in managing data structures efficiently.
Tip: Utilize dynamic arrays for scenarios where the size or values of an array may vary during program execution.
Streamlining Array Operations with Loops
To streamline array operations and reduce repetitive code, leverage loops for efficient processing. Using a loop, such as a for loop, allows you to iterate through array elements seamlessly. By iterating over array elements, you can perform operations uniformly across all values within the array.
Tip: Implement loops to iterate through arrays and perform operations, reducing code redundancy and enhancing maintainability.
Curiosity Question: How can multi-dimensional arrays enhance data representation and processing in complex scenarios?
By exploring these advanced insights into arrays, you can elevate your proficiency in working with array data structures effectively. Ready to unravel more complexities of arrays in programming? Let's continue exploring in the upcoming lessons!
Additional Resources for Arrays
- Arrays in Java - GeeksforGeeks
- Understanding Arrays in Programming - Codecademy
- Introduction to Arrays - Oracle Documentation
Explore these resources to deepen your understanding of arrays and how to effectively work with them in programming languages like Java. Happy learning! 📚💻
Practice
Task
Task: Create a program that reverses an array of integers.
Task: Implement a program that counts the number of vowels in a string.
Task: Write a method that checks if a string is an anagram of another.
Task: Implement a method to merge two arrays into one.
Task: Write a program that finds the maximum value in an array of numbers.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
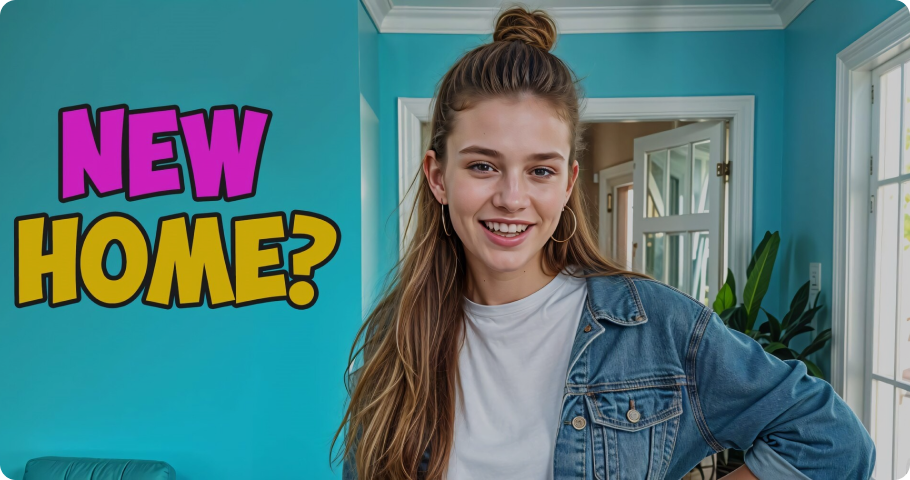
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.