PHP Basics
PHP is a widely-used server-side scripting language designed for web development. It enables the creation of dynamic and interactive websites. Created by Rasmus Lerdorf in 1993, PHP stands for Hypertext Preprocessor, and it's open-source, meaning it’s free to use and modify. PHP allows web developers to interact with databases, manage content, and create dynamic web pages with ease.
Lets Go!
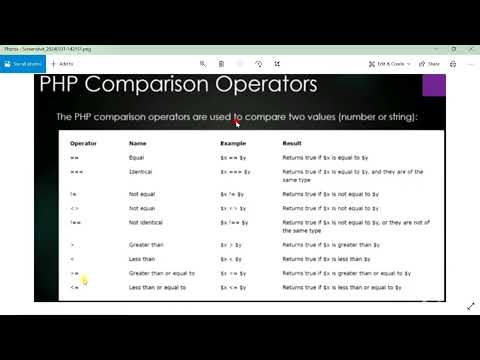
PHP Basics
Level 2
PHP is a widely-used server-side scripting language designed for web development. It enables the creation of dynamic and interactive websites. Created by Rasmus Lerdorf in 1993, PHP stands for Hypertext Preprocessor, and it's open-source, meaning it’s free to use and modify. PHP allows web developers to interact with databases, manage content, and create dynamic web pages with ease.
Get Started 🍁PHP Basics
PHP is a widely-used server-side scripting language designed for web development. It enables the creation of dynamic and interactive websites. Created by Rasmus Lerdorf in 1993, PHP stands for Hypertext Preprocessor, and it's open-source, meaning it’s free to use and modify. PHP allows web developers to interact with databases, manage content, and create dynamic web pages with ease.
Introduction to PHP Operators
Welcome to the course "Introduction to PHP Operators"! In this course, we will explore the essential PHP operators used for performing operations on variables and values. PHP provides a wide range of operators, including arithmetic, assignment, comparison, increment/decrement, logical, and string operators.
Arithmetic Operators: These are used for common arithmetic operations such as addition, subtraction, multiplication, division, modulus, and exponentiation.
Assignment Operators: Used to assign values to variables, such as the equals sign (=), and combined assignment operators like += and *=.
Comparison Operators: These operators compare values or variables to check for equality, inequality, or relative size, such as ==, !=, <, and >.
Curiosity Question: Have you ever wondered how modulus and exponentiation operators work in PHP?
Join us to master PHP operators and learn how to enhance your code’s functionality.
Main Concepts of PHP Operators
-
Arithmetic Operators: These perform basic mathematical operations. For example, addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**).
- Example: Modulus calculates the remainder (e.g., 10 % 3 = 1).
- Example: Exponentiation raises a number to a power (e.g., 10 ** 3 = 1000).
-
Assignment Operators: Used to assign values to variables. Basic assignment is done with
=
. There are also combined operators like+=
,-=
,*=
,/=
, and%=
, which simplify code. -
Comparison Operators: These compare two values to check for equality, inequality, or size differences. For example,
==
,===
,!=
,<
,>
,<=
, and>=
.- The
===
operator checks both value and type. - The
!=
operator checks for inequality.
- The
By mastering these operators, you’ll be able to manipulate data effectively and compare values in your code.
Practical Applications of PHP Operators
Here’s how you can use PHP operators in real-world scenarios:
-
Arithmetic Operators:
- Step 1: Choose numeric values to apply arithmetic operations.
- Step 2: Use operators like
+
,-
,*
,/
,%
,**
. - Step 3: Implement these operators in PHP.
Example:
// Exponentiation $result = 10 ** 3; echo $result; // Output: 1000
-
Assignment Operators:
- Step 1: Assign values to variables with operators like
+=
,-=
,*=
,/=
,%=
. - Step 2: Modify values of existing variables efficiently.
Example:
// Using the += operator $x = 5; $y = 10; $x += $y; echo $x; // Output: 15
- Step 1: Assign values to variables with operators like
-
Comparison Operators:
- Step 1: Compare values using operators like
==
,===
,!=
,<
,>
,<=
,>=
. - Step 2: Use these operators to check if two values are equal or compare them.
Example:
// Comparing values $a = 10; $b = '10'; echo $a === $b; // Output: false
- Step 1: Compare values using operators like
Experiment with these operators in your own code to strengthen your understanding of PHP operators.
Test your Knowledge
What is the purpose of PHP operators?
What is the purpose of PHP operators?
Advanced Insights into PHP Operators
Let’s take a deeper dive into how operators work in PHP and how you can use them for more complex operations:
Arithmetic Operators:
Arithmetic operators are critical for performing mathematical tasks in PHP. Understanding modulus (remainder) and exponentiation (power) will allow you to tackle more advanced calculations.
Tip: Use Exponentiation for Large Calculations
When performing power calculations, use the **
operator. For example, 10 ** 3
results in 1000.
Assignment Operators:
Assignment operators allow you to modify values of variables efficiently. For example, +=
is shorthand for x = x + y
.
Tip: Use Compound Assignment to Simplify Code
By using operators like +=
, -=
and *=
, you can make your code more concise and easier to maintain.
Comparison Operators:
PHP’s comparison operators are useful for making decisions in your code. The ==
operator checks value equality, while ===
ensures both value and type are identical.
Expert Tip: Understand ===
vs. ==
===
checks both the value and type of variables, while ==
only compares the value.
By mastering these operators and applying them in practical situations, you’ll be able to optimize your PHP code for performance and efficiency.
Practice
Task
Task: Write a PHP script that adds two numbers and displays the result.
Task: Create a PHP program to subtract two numbers and display the result.
Task: Use the modulus operator in PHP to find the remainder of 15 divided by 4.
Task: Write a PHP script that compares two variables using ===
and ==
and displays the results.
Task: Create a PHP program that multiplies two numbers and displays the result.
Task: Implement a PHP script that checks if a number is greater than another using comparison operators.
Task: Write a PHP script using the +=
operator to add values to an existing variable.
Task: Write a PHP program that uses *=
to multiply a variable by another value.
Task: Create a PHP script to compare two strings and check if they are equal.
Task: Use the **
operator to calculate the square of a number in PHP.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
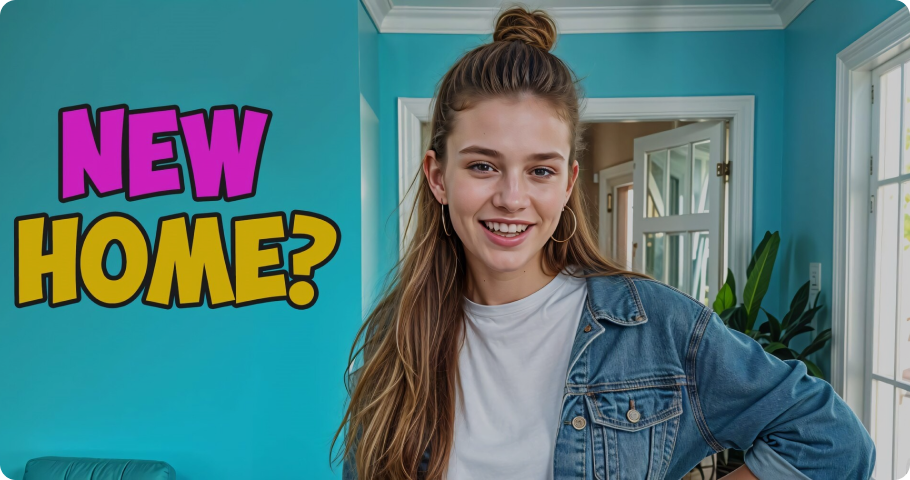
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.