Arrays and Collections
PHP arrays are versatile data structures that allow you to store, organize, and manipulate multiple values in a single variable. Arrays form the backbone of efficient data management in PHP, enabling tasks like grouping related data, creating dynamic lists, and accessing elements with ease.
Lets Go!
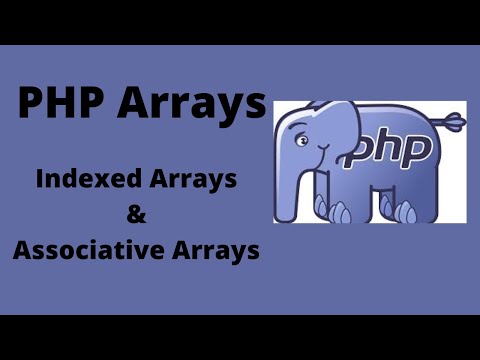
Arrays and Collections
Level 4
PHP arrays are versatile data structures that allow you to store, organize, and manipulate multiple values in a single variable. Arrays form the backbone of efficient data management in PHP, enabling tasks like grouping related data, creating dynamic lists, and accessing elements with ease.
Get Started πArrays and Collections
PHP arrays are versatile data structures that allow you to store, organize, and manipulate multiple values in a single variable. Arrays form the backbone of efficient data management in PHP, enabling tasks like grouping related data, creating dynamic lists, and accessing elements with ease.
Introduction to PHP Arrays
Welcome to the 'Introduction to PHP Arrays' course! Arrays are one of the most powerful features in PHP, offering a convenient way to handle collections of data efficiently. Whether you're working with lists, tables, or nested data structures, arrays are an essential tool in any PHP programmer's toolkit.
In this course, you'll learn how to:
- Create and initialize arrays.
- Work with different types of arrays, including indexed, associative, and multidimensional arrays.
- Use built-in PHP functions to manipulate and iterate through arrays effectively.
By the end of this course, you'll have a strong grasp of how to use arrays to build more dynamic and efficient PHP applications.
Are you ready to enhance your coding skills and explore the flexibility of PHP arrays? Letβs get started!
Main Concepts of PHP Arrays
-
Array Definition:
- Arrays allow you to store multiple values in a single variable, making it easy to group and organize data.
-
Types of Arrays:
- Indexed Arrays: Use numeric indices (0, 1, 2, etc.) to store values sequentially.
- Associative Arrays: Use custom keys (e.g., 'name' => 'Alice') to map keys to values.
- Multidimensional Arrays: Contain nested arrays for hierarchical data structures.
-
Array Syntax:
- Use square brackets
[]
or thearray()
function to define arrays.
// Indexed Array $fruits = ['Apple', 'Banana', 'Cherry']; // Associative Array $person = ['name' => 'John', 'age' => 25]; // Multidimensional Array $products = [ ['id' => 1, 'name' => 'Laptop', 'price' => 899.99], ['id' => 2, 'name' => 'Phone', 'price' => 499.99] ];
- Use square brackets
-
Accessing Array Values:
- Use numeric indices or keys to retrieve specific elements.
echo $fruits[1]; // Output: Banana echo $person['name']; // Output: John
-
Iterating Through Arrays:
- Use loops like
foreach
to process all elements in an array.
foreach ($fruits as $fruit) { echo $fruit . " "; }
- Use loops like
-
Array Manipulation:
- Use built-in functions like
array_push()
,array_merge()
, andarray_keys()
to modify arrays.
array_push($fruits, 'Mango'); $mergedArray = array_merge($fruits, $newArray);
- Use built-in functions like
-
Debugging Arrays:
- Use
print_r()
orvar_dump()
for a detailed view of array structures.
- Use
-
Practical Applications:
- Arrays are used for tasks such as storing user data, managing configurations, and handling API responses.
Practical Applications of PHP Arrays
Task: Managing a Shopping Cart
Learn to use arrays by building a basic shopping cart system:
-
Define a Shopping Cart:
- Create an associative array to store items and their prices.
$cart = [ 'Laptop' => 999.99, 'Headphones' => 49.99, 'Mouse' => 19.99 ];
-
Calculate Total Cost:
- Use a loop to sum up the prices of all items in the cart.
$total = 0; foreach ($cart as $item => $price) { $total += $price; } echo "Total: $total";
-
Add Discounts:
- Add logic to apply a discount to the total if the value exceeds a threshold.
if ($total > 500) { $total *= 0.9; // Apply a 10% discount } echo "Discounted Total: $total";
-
Enhance Functionality:
- Extend the cart to include quantities and calculate total cost dynamically.
$cart = [ ['item' => 'Laptop', 'price' => 999.99, 'quantity' => 1], ['item' => 'Mouse', 'price' => 19.99, 'quantity' => 2] ]; $total = 0; foreach ($cart as $product) { $total += $product['price'] * $product['quantity']; } echo "Final Total: $total";
Try implementing these tasks to gain hands-on experience with arrays!
Test your Knowledge
Which type of array uses numeric indices?
Which type of array uses numeric indices?
Advanced Insights into PHP Arrays
-
Multidimensional Arrays:
- Store complex, hierarchical data structures such as tables or nested lists.
$teams = [ 'Team A' => ['Alice', 'Bob'], 'Team B' => ['Charlie', 'Dave'] ]; echo $teams['Team A'][0]; // Output: Alice
-
Sorting Arrays:
- Use functions like
sort()
,ksort()
, andusort()
to organize data.
sort($fruits); // Sorts alphabetically
- Use functions like
-
Filtering Arrays:
- Use
array_filter()
to remove unwanted values.
$prices = [10, 50, 100]; $filtered = array_filter($prices, function($price) { return $price > 20; });
- Use
-
Array Mapping:
- Apply transformations to each array element with
array_map()
.
$prices = [10, 20, 30]; $doubled = array_map(fn($price) => $price * 2, $prices);
- Apply transformations to each array element with
Additional Resources for PHP Arrays
- PHP Manual on Arrays: PHP.net
- Array Functions in PHP: PHP Array Functions
- Video Tutorials: PHP Arrays Explained
Explore these resources to deepen your understanding of arrays!
Practice
Task
Task: Create an indexed array of your favorite fruits and display each item using a loop.
Task: Define an associative array for a library catalog with book titles and authors.
Task: Write a PHP script to calculate the total cost of items in an associative array.
Task: Create a multidimensional array representing a school timetable and access a specific class time.
Task: Use the array_filter
function to find items in an array greater than a specific value.
Task: Sort an array of numbers in ascending order using the sort
function.
Task: Map an array of prices to apply a 10% discount using array_map
.
Task: Combine two arrays into one using array_merge
and display the result.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
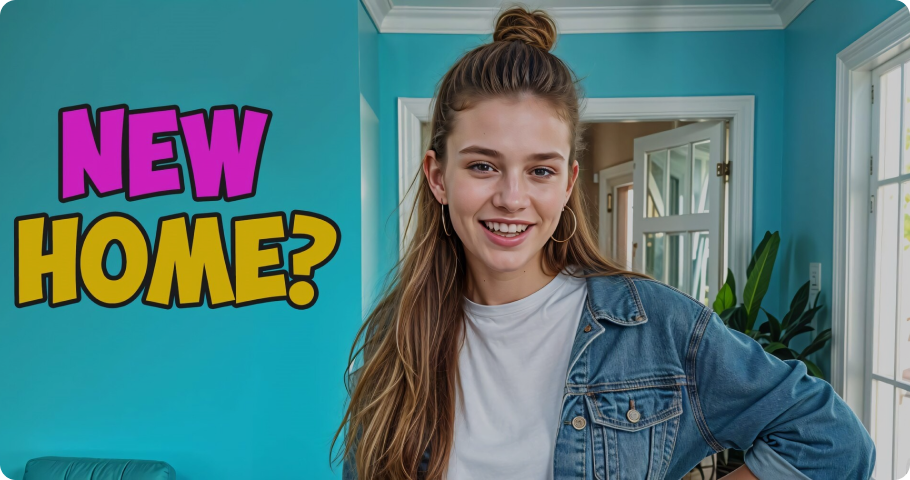
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.