Ruby Syntax and Data Types
Ruby is known for its clean, human-readable syntax. It is dynamically typed, meaning variables don’t need to be declared with a type. Ruby also provides a rich set of built-in data types that allow for efficient data manipulation and storage.
Lets Go!
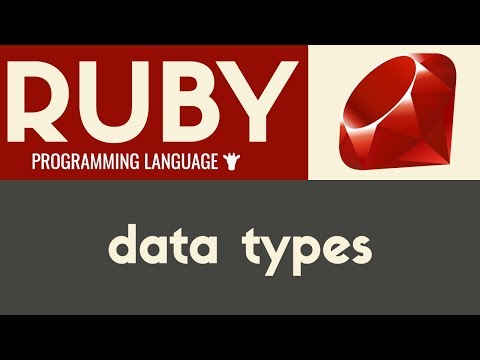
Ruby Syntax and Data Types
Level 2
Ruby is known for its clean, human-readable syntax. It is dynamically typed, meaning variables don’t need to be declared with a type. Ruby also provides a rich set of built-in data types that allow for efficient data manipulation and storage.
Get Started 🍁Ruby Syntax and Data Types
Ruby is known for its clean, human-readable syntax. It is dynamically typed, meaning variables don’t need to be declared with a type. Ruby also provides a rich set of built-in data types that allow for efficient data manipulation and storage.
Ruby Syntax and Data Types
In Ruby, the syntax is designed to be simple and intuitive. Understanding the core data types and syntax rules will help you write clean, readable, and efficient Ruby code. Ruby is dynamically typed, meaning you don't need to explicitly declare variable types. It automatically determines the data type based on the assigned value.
In this lesson, you will:
- Learn about basic Ruby syntax, including variable declarations, methods, and operators.
- Understand Ruby’s core data types such as numbers, strings, arrays, and hashes.
- Discover how to manipulate and work with different data types.
Main Concepts in Ruby Syntax and Data Types
-
Basic Syntax:
- Ruby does not require semicolons at the end of statements.
- Variable names in Ruby are case-sensitive and start with a letter or an underscore.
my_variable = 10 puts my_variable
-
Data Types in Ruby:
- Numbers: Ruby supports integers, floating-point numbers, and complex numbers.
num1 = 42 # Integer num2 = 3.14 # Float num3 = 2 + 3i # Complex
- Strings: Strings are enclosed in single or double quotes.
str1 = 'Hello' str2 = "Ruby World" puts str1 + ' ' + str2
- Symbols: Symbols are immutable and unique identifiers, often used as keys in hashes.
symbol = :ruby_symbol
- Arrays: Arrays are ordered collections of data. They can store multiple data types.
arr = [1, 'apple', 3.14] puts arr[1] # Output: 'apple'
- Hashes: Hashes are collections of key-value pairs.
hash = { 'name' => 'Alice', 'age' => 25 } puts hash['name'] # Output: 'Alice'
-
Variable Assignment:
- Ruby uses dynamic typing, so you don’t need to declare a variable’s type.
age = 25 # Integer name = 'John' # String
-
Operators:
- Ruby provides various operators for arithmetic, comparison, and logic.
sum = 5 + 3 # Addition is_equal = (5 == 5) # Comparison is_true = true && false # Logical
-
Conditional Statements:
- Ruby uses
if
,else
, andelsif
for conditional branching.
if age >= 18 puts 'Adult' else puts 'Minor' end
- Ruby uses
Practical Applications of Ruby Syntax and Data Types
Task: Basic Calculations
- Create a script that performs basic arithmetic operations (addition, subtraction, multiplication, division).
a = 10 b = 5 puts a + b # Addition puts a - b # Subtraction puts a * b # Multiplication puts a / b # Division
Task: String Manipulation
- Write a script that takes user input and prints a greeting message.
print 'Enter your name: ' name = gets.chomp puts 'Hello, ' + name
Task: Create an Array
- Create an array with 5 different data types and print the second element.
arr = [42, 'Ruby', 3.14, true, nil] puts arr[1] # Output: 'Ruby'
Task: Use a Hash
- Create a hash with keys for
name
,age
, andcity
. Print the value associated with the keyage
.person = { 'name' => 'Alice', 'age' => 25, 'city' => 'New York' } puts person['age'] # Output: 25
Test your Knowledge
Which of the following is the correct syntax for declaring a string in Ruby?
Which of the following is the correct syntax for declaring a string in Ruby?
Advanced Insights into Ruby Syntax and Data Types
-
Dynamic Typing in Ruby:
- Ruby’s dynamic typing system allows for flexibility in variable assignment. This can speed up development but requires careful attention to avoid type errors during runtime.
- Ruby also supports type coercion (e.g., converting strings to integers).
-
Strings in Ruby:
- Ruby provides powerful string manipulation methods like
gsub
,split
, andupcase
for working with strings.
str = 'Hello World' puts str.upcase # Output: 'HELLO WORLD' puts str.split(' ') # Output: ['Hello', 'World']
- Ruby provides powerful string manipulation methods like
-
Hash as a Dictionary:
- Hashes are used frequently as dictionaries in Ruby. You can use symbols or strings as keys.
hash = { :name => 'John', :age => 30 } puts hash[:name] # Output: 'John'
-
Multidimensional Arrays:
- Ruby supports arrays of arrays (multidimensional arrays), useful for matrix operations or complex data structures.
matrix = [[1, 2], [3, 4], [5, 6]] puts matrix[1][0] # Output: 3
Additional Resources for Ruby Syntax and Data Types
- Official Ruby Documentation: Ruby Docs
- Learn Ruby the Hard Way: Learn Ruby
- Ruby on Rails Guides: Rails Guides
These resources provide further information about Ruby’s syntax and data types, as well as real-world applications of these concepts.
Practice
Task
Task: Create a script that performs basic arithmetic operations (addition, subtraction, multiplication, division).
Task: Write a Ruby script that asks for the user's name and age and then prints a message with that information.
Task: Create an array with at least 3 different data types and print the first and last elements.
Task: Create a hash to store information about a book (title, author, year) and print the title.
Task: Create a script that takes a string and prints it in uppercase and lowercase.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
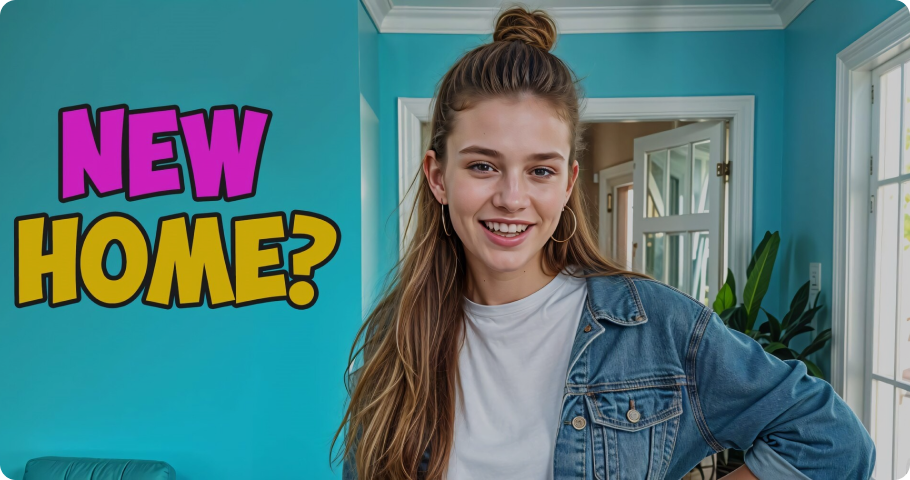
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.