Control Flow: Conditionals and Loops
Control flow statements are crucial for making decisions and repeating tasks in Ruby. Conditionals like `if`, `else`, `elsif`, and loops like `while`, `for`, and `each` enable you to control the flow of your program based on different conditions and iterations.
Lets Go!
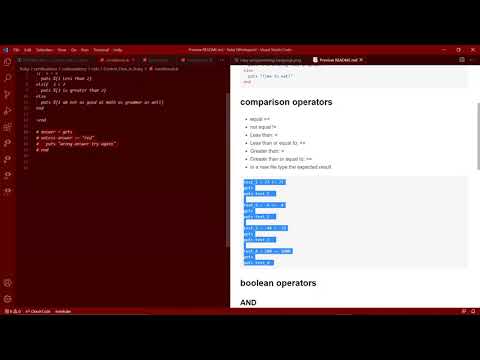
Control Flow: Conditionals and Loops
Level 3
Control flow statements are crucial for making decisions and repeating tasks in Ruby. Conditionals like `if`, `else`, `elsif`, and loops like `while`, `for`, and `each` enable you to control the flow of your program based on different conditions and iterations.
Get Started ๐Control Flow: Conditionals and Loops
Control flow statements are crucial for making decisions and repeating tasks in Ruby. Conditionals like `if`, `else`, `elsif`, and loops like `while`, `for`, and `each` enable you to control the flow of your program based on different conditions and iterations.
Control Flow: Conditionals and Loops
In Ruby, control flow structures allow you to execute different code depending on certain conditions or iterate over collections of data. Mastering conditionals and loops is key to writing dynamic programs that respond to different inputs.
In this lesson, you will learn:
- How to use conditional statements to make decisions in your programs.
- How to loop through data with various types of loops.
- The differences between
if
statements,case
statements, and different looping mechanisms in Ruby.
Main Concepts in Ruby: Control Flow
-
If, Else, Elsif Statements:
- The most common control flow structure in Ruby.
if
evaluates an expression and executes the code block if the condition is true.else
handles cases when the condition is false.elsif
allows checking multiple conditions.
age = 18 if age >= 18 puts 'Adult' elsif age < 18 puts 'Minor' else puts 'Invalid age' end
- The most common control flow structure in Ruby.
-
Case Statements:
- Rubyโs
case
statement is a clean way to handle multiple conditions. Itโs often used when you have many options to check.
grade = 'A' case grade when 'A' puts 'Excellent' when 'B' puts 'Good' when 'C' puts 'Average' else puts 'Invalid grade' end
- Rubyโs
-
While Loop:
- A
while
loop runs as long as the specified condition is true.
count = 0 while count < 5 puts count count += 1 end
- A
-
For Loop:
- The
for
loop is used to iterate over a collection of values, such as an array or range.
for i in 1..5 puts i end
- The
-
Each Loop:
- Rubyโs
each
method is an elegant way to loop through collections like arrays or hashes.
arr = [1, 2, 3, 4] arr.each do |num| puts num end
- Rubyโs
Practical Applications of Ruby Control Flow
Task: Determine Voting Eligibility
- Write a Ruby script that checks if a user is eligible to vote based on their age.
print 'Enter your age: ' age = gets.chomp.to_i if age >= 18 puts 'You are eligible to vote.' else puts 'You are not eligible to vote.' end
Task: Calculate Even or Odd Numbers
- Create a program that takes a number as input and checks if itโs even or odd.
print 'Enter a number: ' num = gets.chomp.to_i if num % 2 == 0 puts 'Even' else puts 'Odd' end
Task: Display Grade Based on Score
- Write a program using a
case
statement to print a grade based on the score.print 'Enter score: ' score = gets.chomp.to_i case score when 90..100 puts 'A' when 80..89 puts 'B' when 70..79 puts 'C' else puts 'F' end
Task: Loop through an Array
- Use a
for
loop to display the elements of an array.fruits = ['apple', 'banana', 'cherry'] for fruit in fruits puts fruit end
Task: Sum Elements in an Array
- Use an
each
loop to find the sum of all elements in an array.numbers = [1, 2, 3, 4, 5] sum = 0 numbers.each do |num| sum += num end puts sum # Output: 15
Test your Knowledge
Which of the following is the correct way to check multiple conditions in Ruby?
Which of the following is the correct way to check multiple conditions in Ruby?
Advanced Insights into Ruby Control Flow
-
Efficient Looping:
- Ruby's
each
loop is more efficient and readable compared to traditionalfor
loops, especially when working with collections like arrays and hashes. - You can also use
each_with_index
to iterate with index in arrays.
arr = ['a', 'b', 'c'] arr.each_with_index do |value, index| puts "#{index}: #{value}" end
- Ruby's
-
Infinite Loops:
- Be cautious when using
while
loops, as they can lead to infinite loops if the condition is always true.
loop do puts 'This will run forever' end
- Be cautious when using
-
Using
next
andbreak
:next
allows you to skip to the next iteration of a loop, whilebreak
allows you to exit a loop prematurely.
(1..5).each do |i| next if i == 3 puts i end # Output: 1, 2, 4, 5
-
Combining Conditionals with Loops:
- You can combine control flow statements to solve complex problems, such as filtering values during iteration.
numbers = [1, 2, 3, 4, 5] numbers.each do |num| break if num == 4 puts num end
Additional Resources for Ruby Control Flow
- Ruby Control Flow Documentation: Ruby Docs
- Ruby Loops and Iterators: Ruby Iterators
- Ruby: The Official Guide: Ruby Guides
Explore these resources to understand more about Ruby's control flow mechanisms and to practice using them in your programs.
Practice
Task
Task: Write a script that asks for the userโs age and prints whether they are eligible to vote.
Task: Create a program to print all the even numbers from 1 to 20.
Task: Write a program that asks for a number and prints 'Even' or 'Odd'.
Task: Use a case
statement to check and print the corresponding season based on the month of the year.
Task: Create a loop that prints the square of numbers from 1 to 10.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
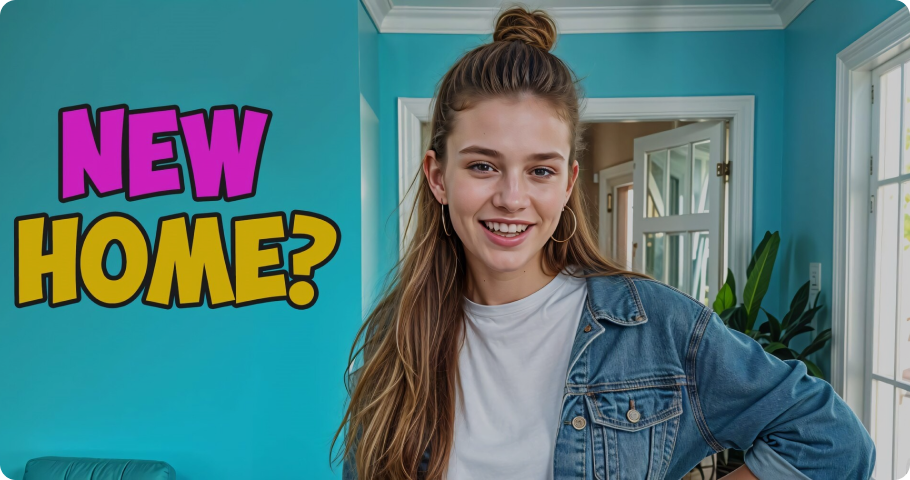
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.