Methods in Ruby
Methods in Ruby are blocks of code that allow you to organize your program into reusable, modular pieces. They can take parameters, return values, and be invoked multiple times, which makes your code more organized and efficient.
Lets Go!
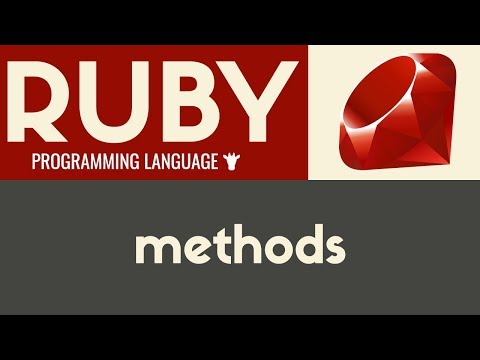
Methods in Ruby
Level 4
Methods in Ruby are blocks of code that allow you to organize your program into reusable, modular pieces. They can take parameters, return values, and be invoked multiple times, which makes your code more organized and efficient.
Get Started 🍁Methods in Ruby
Methods in Ruby are blocks of code that allow you to organize your program into reusable, modular pieces. They can take parameters, return values, and be invoked multiple times, which makes your code more organized and efficient.
Introduction to Methods in Ruby
Welcome to the "Introduction to Methods in Ruby" course at Draft Academy! My name is Mike, and I will be your guide through this tutorial. In this course, we will explore the fundamental concept of methods in Ruby programming.
Methods in Ruby are powerful blocks of code designed to perform specific tasks or functions. By encapsulating code within a method, we can easily reuse it throughout our programs, enhancing code organization and efficiency. In this course, we will cover the basics of creating and utilizing methods in Ruby.
Have you ever wondered how to streamline your code and make it more modular? Well, methods are the solution! By the end of this course, you will have a solid understanding of how methods work, how to pass information to them, and how they can return information back to you.
Join me as we delve into the world of methods in Ruby and discover how they can elevate your programming skills. Get ready to enhance your coding knowledge and embark on an exciting journey into the realm of Ruby methods! Are you ready to dive in? Let's get started!
Main Concepts of Methods in Ruby
-
Definition of Methods: A method in Ruby is a block of code that performs a specific task. It allows us to group code that is designed to perform one task and call it from other parts of the program.
-
Creating a Method: To create a method in Ruby, we use the
def
keyword followed by the method name. Everything betweendef
andend
defines the code block of the method. -
Calling a Method: The code inside a method is only executed when the method is called. Calling a method is done by simply typing out the method name. The method execution flow jumps to the method code block, performs the task, and then returns to the main program flow.
-
Parameters in Methods: Methods in Ruby can accept parameters, which are values passed to the method when it is called. Parameters allow flexibility in defining how a method performs its task.
-
Default Parameter Values: To avoid errors when parameters are not provided, default values can be set for parameters in methods. If a parameter is not provided when calling the method, it will use the default value.
-
Error Handling: In Ruby, when passing different data types, such as integers, they may need to be converted to strings before printing them in a statement to avoid errors.
By understanding these main concepts of methods in Ruby, programmers can effectively organize their code, create reusable blocks of functionality, and make their programs more flexible and error-resistant. Further discussions on return types in methods can enhance the understanding of how methods can both receive and return information.
Practical Applications of Methods in Ruby
Writing a Method to Say Hi
- Define a method by typing
def
followed by the method name, for example,say_hi
. - Write the code block inside the method between
def
andend
. - Within the method block, use
puts
to print a message, for example,puts "hello user"
. - Call the method by typing out the method name,
say_hi
, outside the method block to execute it.
Try it out by following these steps in your Ruby program and see the output hello user
.
Passing Parameters to a Method
- After the method name, add open and closed parentheses to accept parameters, for example,
say_hi(name)
. - Define the parameter inside the method block, for example,
puts "hello #{name}"
. - Call the method with the parameter value, for example,
say_hi("Mike")
.
Test this feature by passing a name parameter to the method and observe the customized output.
Setting Default Parameter Values
- Assign default values to parameters inside the method definition, for example,
name="no name"
. - Use default values for parameters when calling the method without providing all arguments, for example,
say_hi
.
Experiment with default values by omitting some parameters when calling the method and observe the output using default values.
Go ahead and try applying these methods in your Ruby code. Don't hesitate to get hands-on experience to fully understand the concepts discussed in this tutorial. If you encounter any issues or have questions, feel free to leave a comment or seek further guidance. Happy coding!
Test your Knowledge
What keyword is used to define a method in Ruby?
What keyword is used to define a method in Ruby?
Advanced Insights into Methods in Ruby
In Ruby, methods play a crucial role in organizing code to perform specific tasks efficiently. By encapsulating code within methods, developers can call them from various parts of a program, passing in information and receiving outputs. Let's delve into some advanced aspects of working with methods in Ruby:
Tips for Naming Methods
When naming methods, opt for descriptive names that clearly indicate the task they perform. This not only makes your code more readable but also helps you understand the purpose of each method at a glance.
Accepting Parameters
Methods can accept parameters, allowing for dynamic behavior based on input. By defining parameters within parentheses after the method name, you can pass information to the method when calling it. Utilize parameters to enhance the flexibility and reusability of your code.
Using Default Values
To handle scenarios where certain parameters might not always be provided, give parameters default values. This ensures that the method can still execute properly even if specific inputs are missing. Default values enhance the robustness of your methods and provide a safety net for various use cases.
Understanding Execution Flow
When calling methods within a program, Ruby follows a specific execution flow. By grasping how methods are executed sequentially, you can better predict the order in which code will run and handle dependencies between different parts of your program.
Curiosity Question:
How can you leverage methods in Ruby to create more complex behaviors, such as implementing algorithms or processing large datasets efficiently?
Dive deeper into the world of Ruby methods by exploring these advanced insights and experimenting with different ways to optimize your code. Keep refining your method-writing skills to become a proficient Ruby developer!
Additional Resources for Methods in Ruby
- Article: Ruby Methods and Parameters
- Tutorial: Ruby Methods Explained
- Book: "The Well-Grounded Rubyist" by David A. Black
Explore these resources to deepen your understanding of methods in Ruby and enhance your programming skills. Happy coding!
Practice
Task
Task: Write a method that accepts an age and returns whether the person is a minor or an adult.
Task: Create a method that calculates the area of a circle given the radius.
Task: Write a method that accepts a string and returns it reversed.
Task: Create a method that accepts a list of names and returns a list of uppercase names.
Task: Write a method that accepts a number and returns its factorial.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
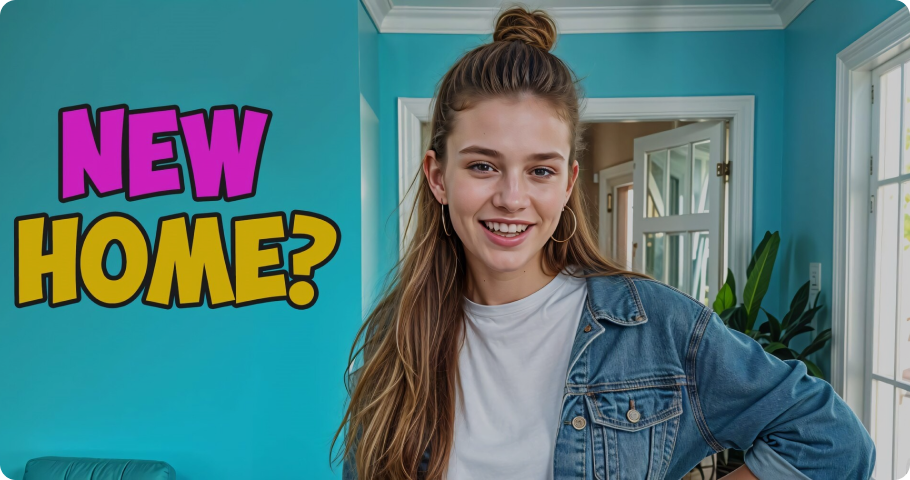
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.