Introduction to Go and Setup
Go (also known as Golang) is a statically typed, compiled programming language designed by Google for simplicity, efficiency, and reliability. It is particularly well-suited for building scalable and high-performance applications, including web servers, networking tools, and more. Setting up Go involves installing the language, setting up the Go workspace, and writing your first Go program.
Lets Go!
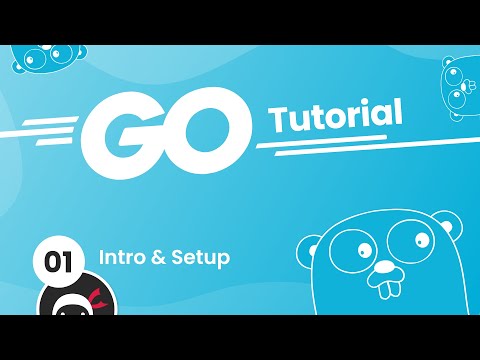
Introduction to Go and Setup
Level 1
Go (also known as Golang) is a statically typed, compiled programming language designed by Google for simplicity, efficiency, and reliability. It is particularly well-suited for building scalable and high-performance applications, including web servers, networking tools, and more. Setting up Go involves installing the language, setting up the Go workspace, and writing your first Go program.
Get Started 🍁Introduction to Go and Setup
Go (also known as Golang) is a statically typed, compiled programming language designed by Google for simplicity, efficiency, and reliability. It is particularly well-suited for building scalable and high-performance applications, including web servers, networking tools, and more. Setting up Go involves installing the language, setting up the Go workspace, and writing your first Go program.
Introduction to Go and Setup
Go, designed by Google, is a modern programming language that focuses on simplicity and performance. It is renowned for its simplicity and powerful concurrency model. Whether you're new to Go or transitioning from another language, it's essential to set up your Go environment and understand its basic components.
In this module, you'll learn:
- How to install Go and set up your Go workspace.
- The structure of a Go program.
- Writing and running your first Go program.
- How to manage dependencies and Go modules.
Main Concepts in Go and Setup
-
Installing Go:
- Visit the official Go website Go Downloads and install Go based on your system's operating system (Windows, macOS, or Linux).
- Follow the installation instructions for your platform.
- After installation, verify by running
go version
in the terminal.
-
Setting up the Go Workspace:
- Go uses a workspace-based structure for organizing code. By default, the Go workspace is located at
$HOME/go
. - The workspace contains three directories:
src
(for source code),bin
(for executable binaries), andpkg
(for compiled package objects). - Set the
GOPATH
environment variable to point to the workspace.
- Go uses a workspace-based structure for organizing code. By default, the Go workspace is located at
-
Hello World in Go:
- Once Go is installed, create a
main.go
file and write the following code:
package main import "fmt" func main() { fmt.Println("Hello, Go!") }
- Run the program with the command
go run main.go
.
- Once Go is installed, create a
-
Understanding Go Modules:
- Go modules provide an easy way to manage dependencies in Go projects.
- To create a Go module, run
go mod init <module-name>
in the project directory. - You can add external packages to your project by running
go get <package-name>
.
-
Running and Building Go Programs:
- Use
go run
to compile and run Go programs directly. - Use
go build
to build an executable binary from your Go code.
go build main.go # Creates an executable binary ./main # Runs the binary
- Use
Practical Applications of Go Setup
Task: Install Go and Write a Simple Program
- Install Go on your system and write a simple 'Hello, World!' program.
package main import "fmt" func main() { fmt.Println("Hello, World!") }
- Use
go run main.go
to run your program.
- Use
Task: Create Your First Go Module
- Initialize a Go module in a project directory using
go mod init
.mkdir myproject cd myproject go mod init myproject
Task: Add an External Package to a Go Project
- Use
go get
to install a package from the Go module repository.go get github.com/gorilla/mux
- Import the package in your Go code and use it.
Task: Build and Run a Go Program
- Write a program in
main.go
, build it usinggo build
, and run the executable binary.go build main.go ./main # Executes the binary
Task: Set Up a Go Workspace
- Create a directory structure for your Go workspace with the default
src
,pkg
, andbin
directories.
Test your Knowledge
What is the command used to verify the Go installation?
What is the command used to verify the Go installation?
Advanced Insights into Go Setup
-
Go Modules and Versioning:
- Go modules allow for easy dependency management. They ensure reproducibility and version control for all dependencies used in your project.
- You can check and update dependencies by using
go mod tidy
andgo mod vendor
.
-
Workspace Management:
- While Go used to rely on the
$GOPATH
workspace, Go modules (introduced in Go 1.11) allow projects to exist outside of theGOPATH
directory, offering more flexibility in project management.
- While Go used to rely on the
-
Cross-Compilation:
- Go makes it easy to cross-compile applications for multiple platforms using the
GOOS
andGOARCH
environment variables. This allows you to build executables for different operating systems.
GOOS=linux GOARCH=amd64 go build
- Go makes it easy to cross-compile applications for multiple platforms using the
-
Understanding Go's Build Process:
- When you run
go run
orgo build
, Go automatically compiles your program and any dependencies before running or creating the executable.
- When you run
-
Exploring the Go Documentation:
- Go’s official documentation (https://golang.org/doc/) provides comprehensive guides, tutorials, and references to help you understand the core concepts and advanced features of the language.
Additional Resources for Go Setup and Introduction
- Go Installation Guide: Go Documentation
- Go Modules Documentation: Go Modules
- Go Playground: Go Online Playground
Check out these resources to get started with Go and dive deeper into its features.
Practice
Task
Task: Write a Go program that prints your name and age to the console.
Task: Create a Go project with a module and install a package such as github.com/gin-gonic/gin
.
Task: Build a Go program and run the executable binary after using go build
.
Task: Set up a Go workspace and organize your Go files into separate directories.
Task: Create a Go program that takes user input and prints it back to the console.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
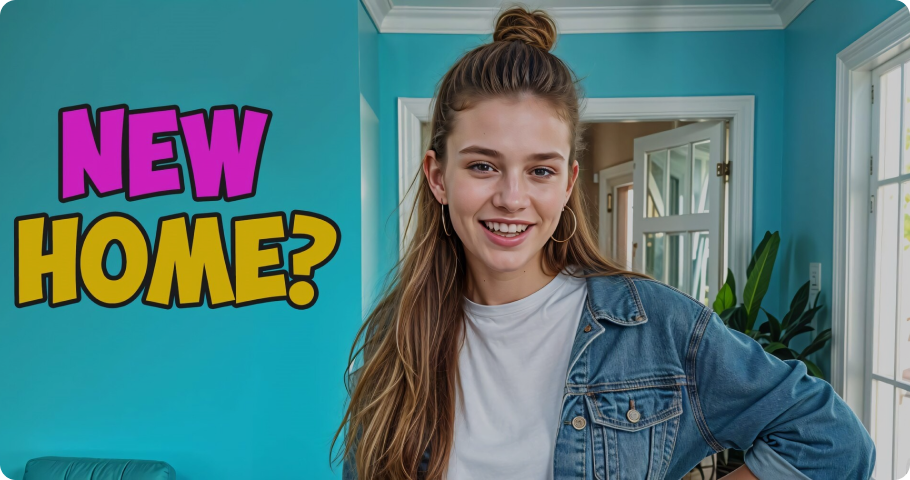
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.