Go Syntax and Data Types
Go has a clean and easy-to-read syntax, making it ideal for both beginners and experienced programmers. In this module, you will learn about Go's syntax rules, including how to define variables, constants, and the basic data types available in Go. Understanding Go’s syntax and data types is essential for writing efficient, reliable programs.
Lets Go!
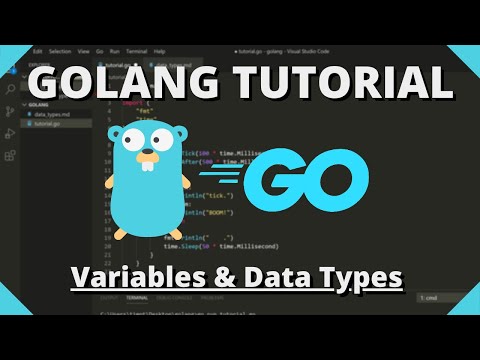
Go Syntax and Data Types
Level 2
Go has a clean and easy-to-read syntax, making it ideal for both beginners and experienced programmers. In this module, you will learn about Go's syntax rules, including how to define variables, constants, and the basic data types available in Go. Understanding Go’s syntax and data types is essential for writing efficient, reliable programs.
Get Started 🍁Go Syntax and Data Types
Go has a clean and easy-to-read syntax, making it ideal for both beginners and experienced programmers. In this module, you will learn about Go's syntax rules, including how to define variables, constants, and the basic data types available in Go. Understanding Go’s syntax and data types is essential for writing efficient, reliable programs.
Go Syntax and Data Types
Go is known for its simple and readable syntax, which allows developers to write efficient programs quickly. Whether you're defining basic variables or using complex data types like slices and maps, understanding Go’s syntax and data types is fundamental to programming in Go.
In this module, you'll learn:
- How to define variables and constants in Go.
- The basic data types available in Go.
- How to use type inference and type conversion.
- The syntax rules for writing Go programs, including functions, conditionals, and loops.
Main Concepts in Go Syntax and Data Types
-
Defining Variables:
- In Go, variables are defined using the
var
keyword. Variables must be declared with a type.
var age int = 30 var name string = "John"
- You can also use shorthand declaration
:=
when the type is inferred.
age := 30 name := "John"
- In Go, variables are defined using the
-
Basic Data Types:
- Go supports several basic data types, including:
- int: Signed integers of varying sizes (e.g.,
int8
,int16
,int32
,int64
) - float32 and float64: Floating-point numbers
- string: A sequence of characters
- bool: Represents true or false values
- int: Signed integers of varying sizes (e.g.,
- Example:
var isActive bool = true var price float64 = 12.99
- Go supports several basic data types, including:
-
Constants:
- Constants in Go are declared using the
const
keyword and cannot be modified after initialization.
const pi float64 = 3.14159 const greeting = "Hello, World!"
- Constants in Go are declared using the
-
Type Inference:
- Go allows for type inference when declaring variables. If the type is not explicitly specified, Go infers the type based on the assigned value.
var number = 10 // Go infers 'number' as int
-
Type Conversion:
- Go is a statically typed language, so explicit type conversion is required when working with different types.
var x int = 10 var y float64 = float64(x) // Convert int to float64
Practical Applications of Go Syntax and Data Types
Task: Write a Go Program to Declare Variables
- Create a program that declares variables of different types and prints them to the console.
var age int = 25 var name string = "Alice" fmt.Println(age, name)
Task: Create and Use Constants
- Define a constant for the value of Pi and print it.
const pi float64 = 3.14159 fmt.Println(pi)
Task: Type Inference Example
- Write a program that demonstrates Go’s type inference.
age := 30 height := 5.9 fmt.Println(age, height)
Task: Type Conversion Example
- Convert an integer to a float and print the result.
var num int = 100 var floatNum float64 = float64(num) fmt.Println(floatNum)
Task: Boolean and String Variables
- Write a program that declares a boolean variable and a string variable, then prints them to the console.
var isActive bool = true var greeting string = "Welcome!" fmt.Println(isActive, greeting)
Test your Knowledge
Which of the following is the correct way to declare a variable in Go?
Which of the following is the correct way to declare a variable in Go?
Advanced Insights into Go Syntax and Data Types
-
Custom Types:
- Go allows you to create custom types using the
type
keyword. This can be useful for creating more meaningful names for existing types.
type Age int var age Age = 30
- Go allows you to create custom types using the
-
Pointers:
- Pointers in Go allow you to store the memory address of a variable, enabling efficient memory management.
- You can create pointers using the
&
operator and dereference them with*
.
var num int = 58 var ptr *int = &num fmt.Println(*ptr) // Dereference to get the value
-
Zero Values:
- Go provides default values (zero values) for variables that are not initialized. For example, an uninitialized
int
will default to0
, and an uninitializedstring
will default to an empty string.
- Go provides default values (zero values) for variables that are not initialized. For example, an uninitialized
-
Arrays and Slices:
- Go supports both arrays and slices. Arrays have a fixed size, while slices are dynamic.
var arr [3]int = [3]int{1, 2, 3} var slice []int = []int{1, 2, 3}
-
Maps:
- Maps are Go's built-in associative arrays, allowing you to store key-value pairs.
var userDetails map[string]string userDetails = make(map[string]string) userDetails["name"] = "John"
Additional Resources for Go Syntax and Data Types
- Go Programming Language Documentation: Go Documentation
- Go by Example: Go by Example
- Go Wiki - Data Types: Go Wiki
These resources will help you gain a deeper understanding of Go's syntax and data types and improve your Go programming skills.
Practice
Task
Task: Declare and initialize variables of different types (int, float64, string, and bool), then print their values.
Task: Create a program to convert a floating-point number to an integer and print both the original and converted values.
Task: Write a program that declares a constant and prints its value.
Task: Create a map of key-value pairs representing student names and grades, then retrieve and print the grade for a specific student.
Task: Write a program that demonstrates Go’s type inference and prints the inferred types.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
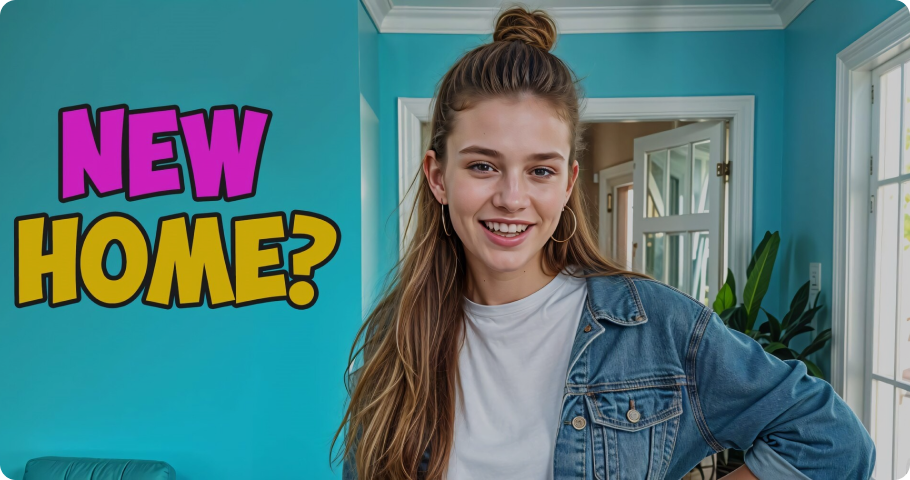
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.