Functions in Go
Functions are the building blocks of Go programs. They allow you to organize and modularize code, making it reusable, maintainable, and easier to understand. In Go, functions can have parameters, return values, and can be assigned to variables or passed as arguments. Understanding functions is essential for writing clean, efficient Go programs.
Lets Go!
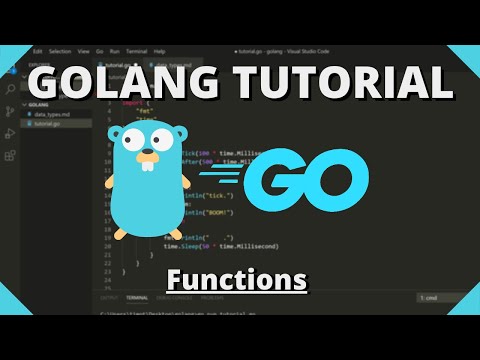
Functions in Go
Level 4
Functions are the building blocks of Go programs. They allow you to organize and modularize code, making it reusable, maintainable, and easier to understand. In Go, functions can have parameters, return values, and can be assigned to variables or passed as arguments. Understanding functions is essential for writing clean, efficient Go programs.
Get Started 🍁Functions in Go
Functions are the building blocks of Go programs. They allow you to organize and modularize code, making it reusable, maintainable, and easier to understand. In Go, functions can have parameters, return values, and can be assigned to variables or passed as arguments. Understanding functions is essential for writing clean, efficient Go programs.
Functions in Go
Functions are essential in Go as they encapsulate a set of instructions to perform specific tasks. You can define functions with parameters, return values, and even variadic parameters. In Go, functions are first-class citizens, meaning they can be assigned to variables, passed around, and even returned from other functions. In this module, you'll learn how to define and call functions in Go.
In this lesson, you'll learn how to:
- Define simple functions in Go.
- Work with functions that take parameters and return values.
- Understand multiple return values in Go functions.
- Explore anonymous functions and function literals.
Main Concepts of Functions in Go
-
Defining Functions:
- Functions in Go are defined using the
func
keyword, followed by the function name and a list of parameters in parentheses. The return type is specified after the parameters.
func greet(name string) { fmt.Println("Hello, " + name) }
- Functions in Go are defined using the
-
Calling Functions:
- Functions are called by using the function name followed by arguments inside parentheses.
greet("Alice")
-
Return Values:
- Functions can return values to the caller. To return a value, specify the return type after the parameters.
func add(a int, b int) int { return a + b }
-
Multiple Return Values:
- Go supports returning multiple values from a function.
func divide(a, b int) (int, int) { quotient := a / b remainder := a % b return quotient, remainder }
- When calling a function that returns multiple values, you can assign them to separate variables.
q, r := divide(10, 3) fmt.Println(q, r) // Output: 3 1
-
Named Return Values:
- Go allows you to name the return values, which will automatically be used as variables within the function body.
func multiply(a, b int) (product int) { product = a * b return }
-
Variadic Functions:
- Variadic functions allow you to pass a variable number of arguments. Use the
...
syntax in the parameter list.
func sum(nums ...int) int { total := 0 for _, num := range nums { total += num } return total }
- Call the function with any number of arguments.
fmt.Println(sum(1, 2, 3, 4)) // Output: 10
- Variadic functions allow you to pass a variable number of arguments. Use the
-
Anonymous Functions:
- Functions in Go can be anonymous (without a name) and used for inline tasks or assigned to variables.
greet := func(name string) { fmt.Println("Hello, " + name) } greet("Bob")
Practical Applications of Functions in Go
Task: Basic Arithmetic Operations
- Write functions to perform basic arithmetic operations (addition, subtraction, multiplication, division). Each function should take two
int
parameters and return the result.func add(a, b int) int { return a + b } func subtract(a, b int) int { return a - b } func multiply(a, b int) int { return a * b } func divide(a, b int) (int, int) { return a / b, a % b }
Task: Fibonacci Sequence
- Write a function to calculate the nth Fibonacci number using recursion.
func fibonacci(n int) int { if n <= 1 { return n } return fibonacci(n-1) + fibonacci(n-2) }
Task: String Manipulation
- Create a function that takes a string and returns the string reversed.
func reverse(str string) string { result := "" for i := len(str) - 1; i >= 0; i-- { result += string(str[i]) } return result }
Task: Calculate Factorial
- Write a function to calculate the factorial of a number using iteration.
func factorial(n int) int { result := 1 for i := 1; i <= n; i++ { result *= i } return result }
Test your Knowledge
How do you define a function in Go?
How do you define a function in Go?
Advanced Insights into Functions in Go
-
Deferred Function Execution:
- The
defer
keyword is used to ensure that a function call is executed after the surrounding function has finished. Deferred functions are commonly used for cleanup tasks (like closing files).
func writeFile(filename string) { file, err := os.Create(filename) if err != nil { fmt.Println(err) return } defer file.Close() // Ensures file is closed after the function finishes fmt.Fprintln(file, "Hello, Go!") }
- The
-
Closures:
- Closures are functions that capture variables from their surrounding scope. These are useful for creating functions on the fly with custom behavior.
func adder(x int) func(int) int { return func(y int) int { return x + y } } add5 := adder(5) fmt.Println(add5(10)) // Output: 15
-
Function Literals:
- Function literals are anonymous functions that can be defined and invoked inline.
func() { fmt.Println("Hello from function literal") }() // Immediately invoked function literal
Additional Resources for Functions in Go
- Go Functions Documentation: Go Docs - Functions
- Go Variadic Functions: Go Docs - Variadic Functions
These resources will further enhance your understanding of Go's function usage and patterns.
Practice
Task
Task: Write a function that takes a number and returns whether it is even or odd.
Task: Create a function that takes two numbers and returns their maximum value.
Task: Implement a recursive function to calculate the factorial of a number.
Task: Write a function that reverses a string.
Task: Create a function that accepts any number of integers and returns the sum.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
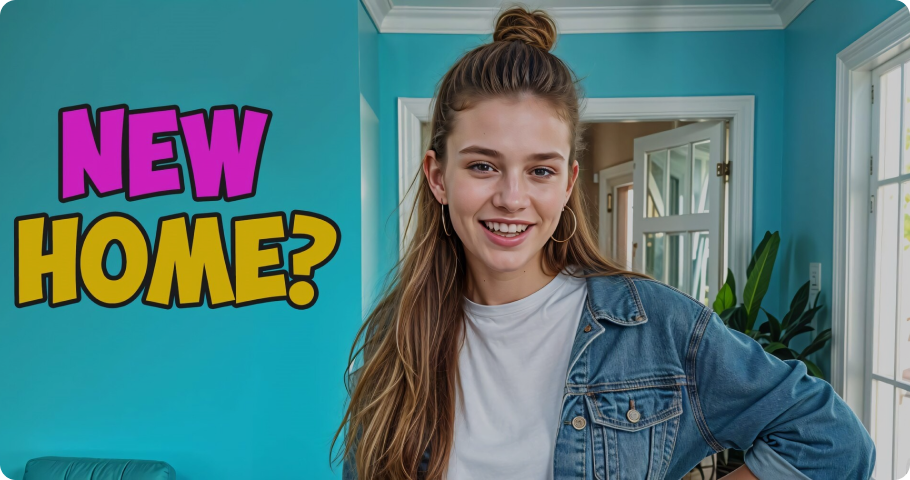
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.