Arrays, Slices, and Maps in Go
Arrays, slices, and maps are the core data structures in Go for working with collections of data. Arrays are fixed-length, while slices provide a more flexible, dynamically-sized alternative. Maps are key-value pairs that allow for efficient lookups and organization of data. Understanding these structures is key to writing efficient Go programs.
Lets Go!
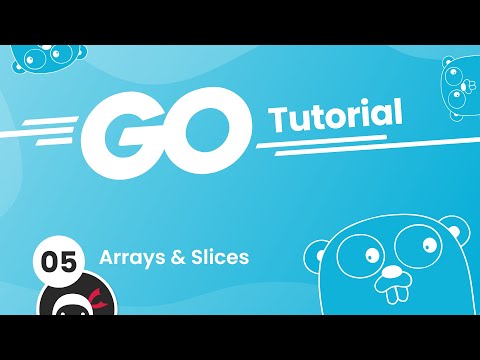
Arrays, Slices, and Maps in Go
Level 5
Arrays, slices, and maps are the core data structures in Go for working with collections of data. Arrays are fixed-length, while slices provide a more flexible, dynamically-sized alternative. Maps are key-value pairs that allow for efficient lookups and organization of data. Understanding these structures is key to writing efficient Go programs.
Get Started 🍁Arrays, Slices, and Maps in Go
Arrays, slices, and maps are the core data structures in Go for working with collections of data. Arrays are fixed-length, while slices provide a more flexible, dynamically-sized alternative. Maps are key-value pairs that allow for efficient lookups and organization of data. Understanding these structures is key to writing efficient Go programs.
Introduction to Arrays and Slices in Go
Welcome to the course on "Introduction to Arrays and Slices in Go"! In this course, we will dive into the fundamentals of working with arrays and slices in the Go programming language.
Background Information
Arrays and slices are essential data structures in Go that allow you to store collections of elements. While arrays have a fixed length and type, slices provide more flexibility by allowing you to add or remove elements dynamically.
Setting Up Arrays
When declaring an array in Go, you specify the length of the array and the type of elements it will contain. Unlike some other programming languages, arrays in Go have a fixed length and cannot be changed once declared.
Setting Up Slices
Slices, on the other hand, can change in length by adding or removing elements. They are built on top of arrays and offer more versatility when working with collections of data.
Course Prerequisites
To get the most out of this course, it is recommended that you have a basic understanding of Go programming concepts such as variable declaration, data types, and basic syntax.
Curiosity Question
Have you ever wondered how to efficiently manage and manipulate collections of data in Go? Join us in this course to explore the world of arrays and slices and unlock the full potential of data handling in Go!
Let's embark on this journey together and deepen our understanding of arrays and slices in Go. Let's get started! 🚀
Main Concepts of Arrays and Slices
-
Arrays
- Arrays are a fixed-length collection of items of the same data type in Go.
- To declare an array, use the
var
keyword followed by the array name, size, and data type within square brackets, then initialize it with values inside curly braces. - Unlike other languages like Python, the length of an array in Go cannot be changed once declared.
- The elements in an array must all be of the same data type.
- You can also use shorthand assignment to create arrays in Go.
- Use the
len
function to get the length of an array.
-
Slices
- Slices, like arrays, hold elements of the same data type, but are more flexible and can change in length.
- To declare a slice, omit the size within square brackets while initializing it with values in curly braces.
- Slices underneath use arrays, but allow flexibility in adding or removing elements.
- You can modify elements within a slice by indexing and assigning new values.
- Use the
append
function to add new elements to a slice, which returns a new slice that needs to be reassigned. - The length of a slice can be changed dynamically, unlike with arrays.
-
Slice Ranges
- Slice ranges are a way to extract a subset of elements from an existing array or slice.
- When using slice ranges, the first position is inclusive, but the second position is exclusive.
- Accessing elements beyond the length of the slice will automatically include all the remaining elements.
- Ranges can be used to create new slices containing specific elements from an existing array or slice.
- Appending new elements to a slice range is possible, as ranges are essentially slices themselves.
Understanding the difference between arrays and slices, as well as how to manipulate them using ranges, is crucial for effective programming in Go. Arrays provide fixed-length storage, while slices offer more flexibility in managing collections of data.
Practical Applications of Arrays and Slices
Arrays and slices are essential data structures in Go that allow you to store collections of values efficiently. Here's how you can work with arrays and slices in your Go programs:
Creating and Initializing Arrays
-
To create an array, declare a variable using the
var
keyword followed by the array name and its type within square brackets.var ages [3]int
-
Initialize the array with values by assigning them using curly braces.
ages = [3]int{20, 25, 30}
-
Remember that arrays have a fixed length and type. You cannot add or remove elements once the array is declared.
Working with Slices
-
To create a slice, omit the length in the square brackets during declaration.
var scores []int
-
Initialize the slice similar to an array using curly braces and values inside.
scores = []int{150, 60}
-
You can update values within an array or slice by accessing the index and assigning a new value.
names[1] = "Luigi" scores[2] = 25
-
Append new elements to a slice using the
append()
function.scores = append(scores, 85)
Slice Ranges
-
Create a range by specifying the indices you want to extract from the original slice.
range1 := names[1:3]
-
Ranges are inclusive of the start index but exclusive of the end index.
// Extracts elements at position 1 and 2 but not 3
-
You can append new values to ranges as well.
range1 = append(range1, "Cooper")
Try It Out
Take the following steps to experiment with arrays, slices, and ranges:
- Declare an array with your favorite numbers and print out its length.
- Create a slice of names containing some of your friends' names and update one of the names.
- Append a new name to the slice and print out the updated slice.
- Experiment with slice ranges to extract subsets of the names slice.
- Append a new value to a range and observe the changes.
Dive into coding and have fun exploring the versatility of arrays, slices, and ranges in Go!
Test your Knowledge
Which of the following statements correctly initializes a slice in Go?
Which of the following statements correctly initializes a slice in Go?
Advanced Insights into Arrays and Slices
Moving beyond basic understanding of arrays and slices, let's delve into some advanced insights to enhance your knowledge:
Arrays:
-
Array Declaration: Arrays in Go are declared using the
var
keyword followed by the array name, size in square brackets, and type. They have a fixed length that cannot be changed once declared. -
Creating Arrays: Arrays can be initialized by specifying the number of elements and their values within curly braces.
-
Shorthand Assignment: Go allows shorthand assignment for arrays where types are inferred automatically without explicit declaration.
Tip:
When dealing with arrays in Go, ensure that the declared length matches the actual number of elements to avoid runtime errors.
Curiosity question:
How does Go handle multi-dimensional arrays compared to other programming languages?
Slices:
-
Dynamic Length: Slices in Go are more flexible than arrays and allow you to change the length by adding or removing elements.
-
Appending Elements: Slices can grow dynamically by appending elements using the
append()
function. Remember to reassign the modified slice back to the variable. -
Manipulating Slices: Changing individual elements or ranges within a slice is possible, providing greater flexibility in data manipulation.
Tip:
When using the range
function with slices, understand that it includes the starting index but excludes the ending index.
Curiosity question:
How does the underlying array structure impact the performance of operations on slices compared to arrays?
These insights into arrays and slices will help you grasp the intricacies of working with structured data in Go, enabling you to write more efficient and robust code. Happy coding!
Additional Resources for Arrays and Slices in Go
Here are some valuable resources to further enhance your understanding of arrays and slices in Go:
- Official Go Documentation on Arrays
- Official Go Documentation on Slices
- Go by Example: Arrays
- Go by Example: Slices
- Arrays and Slices in Go - A Practical Guide
- Understanding Go Arrays and Slices
Dive into these resources to deepen your knowledge and practical skills in working with arrays and slices in Go. Happy learning! 🚀
Practice
Task
Task: Create a slice that holds the first 5 square numbers (1, 4, 9, 16, 25).
Task: Write a program to find the largest number in a slice of integers.
Task: Implement a program that takes a list of words and counts how many times each word appears using a map.
Task: Create a function that combines two slices into one.
Task: Write a program that removes a given key from a map and prints the resulting map.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
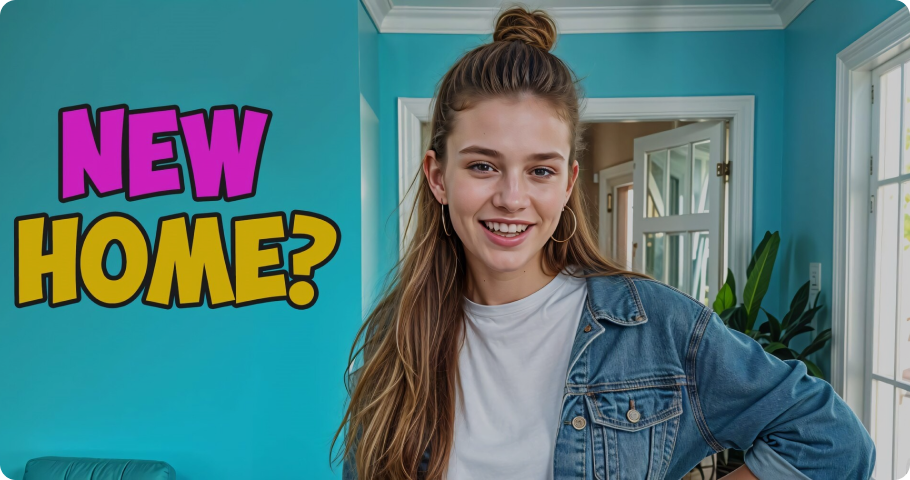
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.