Data Structures and Algorithms
Data structures are essential for storing, organizing, and managing data efficiently. Algorithms leverage these structures to optimize performance and solve complex problems. This course will explore both built-in and user-defined data structures in Python.
Lets Go!
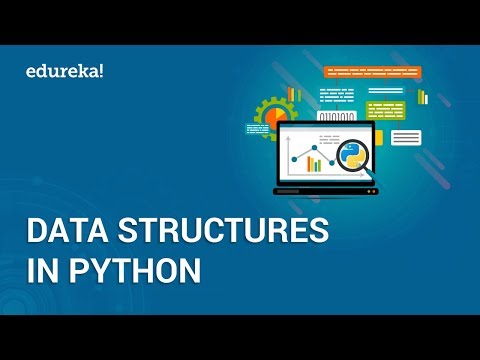
Data Structures and Algorithms
Level 10
Data structures are essential for storing, organizing, and managing data efficiently. Algorithms leverage these structures to optimize performance and solve complex problems. This course will explore both built-in and user-defined data structures in Python.
Get Started 🍁Data Structures and Algorithms
Data structures are essential for storing, organizing, and managing data efficiently. Algorithms leverage these structures to optimize performance and solve complex problems. This course will explore both built-in and user-defined data structures in Python.
Introduction to Data Structures in Python
Welcome to the course "Introduction to Data Structures"! In this course, we will explore the fundamental aspects of data structures in Python and how they play a crucial role in programming.
Data structures are essential for storing, organizing, and managing data efficiently. By using data structures, we can improve access to data and streamline operations, ultimately reducing the time required to solve problems.
During this course, we will delve into various built-in and user-defined data structures in Python, such as lists, dictionaries, tuples, sets, stacks, queues, trees, hashmaps, graphs, and linked lists. These structures are integral for processing and manipulating data in a structured manner.
Have you ever wondered how data can be arranged and accessed seamlessly like setting up devices at your workplace? Data structures in Python enable us to achieve this organization and optimization, making them a fundamental concept in programming.
Get ready to explore the world of data structures in Python and enhance your understanding of how to efficiently work with data. Let's embark on this learning journey together!
Main Concepts of Data Structures
- Data Structures:
- Data structures are used to store, organize, and manage data, providing better access and reducing the time required to solve problems.
- Built-in Data Structures:
- Lists, dictionaries, tuples, and sets are examples of built-in data structures in Python.
- User-defined Data Structures:
- User-defined data structures in Python include stacks, queues, trees, hashmaps, and graphs.
- Lists:
- Lists in Python are used to store data in a sequential manner and can contain heterogeneous data types.
- Elements can be added to lists using
append
,extend
, andinsert
functions. - Data can be accessed and modified using indexes.
- Elements can be deleted using
del
,pop
, andremove
functions. - Sorting, finding index, counting elements, and other operations can be performed on lists.
- Tuples:
- Tuples in Python are similar to lists but are immutable, meaning data cannot be changed once stored.
- Dictionaries:
- Dictionaries hold key-value pairs and are used to store data based on keys for easier access and manipulation.
- Elements can be added, modified, and deleted in dictionaries.
- Sets:
- Sets in Python are unordered collections of unique elements that do not allow duplicate values.
- Elements can be added to sets using the
add
function and operations like union, intersection, difference, and symmetric difference can be performed.
- Stacks:
- Stacks follow the LIFO (Last In First Out) principle where the last element to be added is the first to be removed.
- Elements can be added to stacks using
push
and removed usingpop
.
- Queues:
- Queues follow the FIFO (First In First Out) principle where the first element to be added is the first to be removed.
- Elements can be added to queues using
enqueue
(add) and removed usingdequeue
.
- Trees:
- Trees represent hierarchical structures with a root node and child nodes.
- Nodes are connected by edges, and operations like insert, search, delete can be performed on trees.
- Linked Lists:
- Linked lists are made up of nodes containing data and a pointer to the next node.
- Elements can be inserted, searched, and deleted in linked lists.
- Graphs:
- Graphs consist of vertices (nodes) connected by edges representing relationships.
- Graphs are used to represent relationships and perform calculations like finding the shortest path.
Practical Applications of Data Structures
Step-by-Step Guide to Using Lists in Python:
- Open PyCharm or any Python IDE on your system.
- Create a new Python file and name it "lists".
- Define a list using square brackets, for example:
list1 = []
. - Populate the list with data elements of heterogeneous data types.
- Use the following functions to add elements:
list1.append(2)
to add a single element.list1.extend([2, 0])
to add multiple elements.list1.insert(3, "example")
to insert an element at a specific index.
- Utilize the following functions to delete elements:
del list1[3]
to delete a specific element.list1.pop(4)
to remove and return an element at a specific index.list1.remove(1)
to delete a specific value from the list.
- Access elements using indexes and manipulate the list as needed.
- Demonstrate sorting and finding elements using functions like
sort
,sorted
,index
, andcount
.
By following these steps, you can explore the practical applications of lists in Python and understand how data structures like lists are used for efficient data management.
Try out the steps above in your Python environment to practice using lists effectively. Manipulate the elements, add new data, and explore the functionalities of lists to deepen your understanding of data structures in Python. Embrace hands-on learning to enhance your programming skills and optimize data management processes.
Test your Knowledge
What is the primary purpose of data structures in programming?
What is the primary purpose of data structures in programming?
Advanced Insights into Data Structures
Data structures in Python are essential for efficient data storage and management, allowing for easier access and operations. Understanding the built-in and user-defined data structures is crucial for efficient programming practices.
Built-in Data Structures
Python offers built-in data structures such as lists, dictionaries, tuples, and sets. These structures provide powerful functions for data manipulation and organization. Lists are mutable sequences that can hold heterogeneous data types, while dictionaries store key-value pairs for efficient lookup operations. Tuples are immutable sequences ideal for storing fixed data, and sets are collections of unique elements, useful for mathematical operations.
User-Defined Data Structures
In addition to built-in structures, Python supports user-defined data structures like stacks, queues, trees, hashmaps, graphs, and linked lists. Stacks follow the Last-In-First-Out (LIFO) principle, queues adhere to the First-In-First-Out (FIFO) principle, trees represent hierarchical data structures, linked lists connect nodes sequentially, and graphs depict vertices and edges for complex data relationships.
Tips for Effective Data Structure Usage:
- Understand the principles behind data structures for efficient implementation.
- Choose the right structure based on the nature of the data and the required operations.
- Practice traversing, inserting, and deleting elements in different data structures to enhance proficiency.
- Utilize advanced functions like sorting, searching, and manipulation to optimize data handling.
Expert Advice:
"Mastering data structures is key to becoming a proficient Python programmer. Experiment with different structures, understand their strengths and weaknesses, and leverage them effectively in your projects."
Curiosity Question:
How can data structures like trees and graphs be applied in real-world scenarios to solve complex problems efficiently?
Exploring advanced data structures in Python opens up a vast array of possibilities for organizing and managing data effectively. Dive deeper into each structure, experiment with different functions, and unleash the full potential of Python data handling capabilities. Happy learning!
By delving into the intricacies of data structures, programmers can enhance their proficiency in Python and elevate their programming skills to new heights. Stay curious, keep exploring, and embrace the power of data structures in your coding journey.
Additional Resources for Data Structures
- Python Data Structures - W3Schools: An extensive guide to Python data structures with examples and explanations.
- Data Structures and Algorithms in Python - GeeksforGeeks: A comprehensive collection of articles and tutorials on various data structures in Python.
- Python Data Structures and Algorithms - Real Python: In-depth tutorials and practical examples to learn about data structures in Python.
- Data Structures in Python - TutorialsPoint: Beginner-friendly tutorials on data structures in Python to enhance your understanding.
- Data Structures and Algorithms in Python - Coursera: A specialization course on Coursera to deepen your knowledge of data structures and algorithms in Python.
Practice
Task
Task: Write a Python function that adds two numbers together. Create a unit test to ensure the function works as expected. Use a stack or queue to test multiple cases.
Task: Create a Python program that uses a dictionary to store and retrieve user information. Write unit tests for adding, updating, and deleting user records from the dictionary.
Task: Implement a function that checks if a number is a prime number. Write a unit test to validate the function for various inputs, using a stack or queue to manage test cases.
Task: Write a function that implements a simple stack (using a list) in Python. Implement basic operations such as 'push()', 'pop()', and 'peek()'. Write unit tests to validate each operation.
Task: Create a Python program to demonstrate how a queue can be used to simulate the checkout process in a store. Write unit tests to verify that customers are processed in the correct order.
Task: Implement a linked list that stores integers. Include methods to add and remove elements, as well as to search for a specific number. Write unit tests to verify the linked list functionality.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
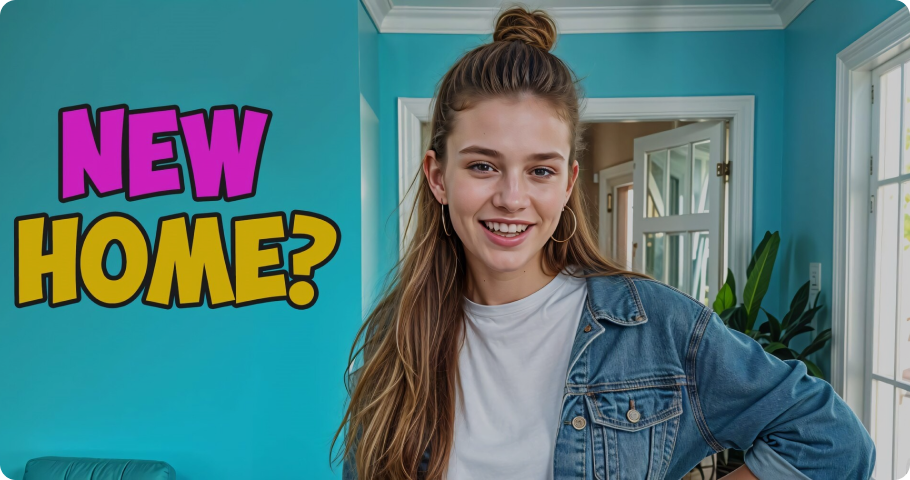
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.