Collections
Understand how to create, modify, and manipulate lists
Introduction to Lists in Python
Welcome to "Introduction to Lists in Python"! In this course, we will dive into the fundamental concept of lists in Python, a versatile data structure that allows you to store multiple items within a single variable.
Have you ever wondered how you can efficiently manage and access lists of data in Python? Well, look no further! Lists are a powerful tool that can enhance your coding skills and streamline your programs.
Throughout this course, we will explore the ins and outs of lists, learning how to add, access, update, and manipulate elements within a list. We will also cover essential functions such as append
, remove
, pop
, insert
, sort
, and more, enabling you to harness the full potential of lists in Python.
Before we get started, make sure you have a basic understanding of Python programming concepts. If you're ready to embark on this exciting journey of mastering lists in Python, let's begin by uncovering the magic behind this essential data structure. What secrets lie within the realms of lists that await your discovery? Join us to find out!
Main Concepts of Lists in Python
-
Lists: A list in Python is used to store multiple items within a single variable. It allows you to store various values in one place, turning a variable into a collection.
-
Accessing Elements: Elements in a list are accessed by their index, starting from zero. To access a specific element, you use square brackets with the index number inside.
-
Updating Elements: The elements in a list can be updated or changed at any point in the program. You can replace an element with a new value simply by assigning a new value to the desired index.
-
Printing Elements: You can print all elements in a list using a for loop that iterates through each item in the list and prints it out.
-
List Functions:
- Append: Add an element to the end of the list using the
append
function. - Remove: Delete a specific value from the list using the
remove
function. - Pop: Remove the last element from the list using the
pop
function. - Insert: Add a value at a specified index in the list using the
insert
function. - Sort: Sort the elements in the list alphabetically using the
sort
function. - Clear: Remove all elements from the list using the
clear
function.
- Append: Add an element to the end of the list using the
By understanding these main concepts of lists in Python, you can efficiently work with collections of data and manipulate them as needed in your programs. Lists provide a flexible way to handle multiple values within a single variable.
Practical Applications of Lists in Python
Let's put our knowledge of lists in Python to use with some practical examples! Follow these steps to manipulate and work with lists in your own Python programs:
-
Creating a List:
- Define a list by enclosing values in square brackets:
food = ['pizza', 'hamburger', 'hot dog', 'spaghetti']
.
- Define a list by enclosing values in square brackets:
-
Accessing Elements:
- Print the entire list:
print(food)
. - Access elements by index (starting from 0):
print(food[0])
will output 'pizza'.print(food[1])
will output 'hamburger'.
- Print the entire list:
-
Updating Elements:
- Change an element in the list:
food[0] = 'sushi'
. - Print the updated list to see the changes.
- Change an element in the list:
-
Displaying all Elements:
- Use a
for
loop to print all elements in the list:for x in food: print(x)
- Use a
-
Using List Functions:
- Append an element to the end of the list:
food.append('ice cream')
. - Remove an element from the list:
food.remove('hot dog')
. - Remove the last element in the list:
food.pop()
. - Insert a value at a specific index:
food.insert(0, 'cake')
. - Sort the list alphabetically:
food.sort()
. - Clear all elements from the list:
food.clear()
.
- Append an element to the end of the list:
Try out these steps in your Python environment to see how lists can be manipulated and utilized effectively in your programs. Remember, hands-on practice is key to mastering Python lists! 🐍✨
So go ahead and write some code using lists, and feel free to ask any questions or share your experiences in the comments below. Happy coding! 🚀
Keep Learning and Exploring
If you found this guide helpful, don't forget to show your support by liking the video and subscribing for more Python tutorials. Let's keep learning together and becoming Python pros! 💪📚
Test your Knowledge
What is the purpose of the append
function in Python?
What is the purpose of the append
function in Python?
Advanced Insights into Lists in Python
Now that we have a basic understanding of lists in Python, let's delve into some more advanced insights to enhance our knowledge further.
Tips for Efficient List Handling
- List Indexing: Remember that indexes in Python start from 0. When accessing elements in a list, always keep this in mind to avoid errors.
- Updating Elements: Lists are mutable, meaning you can update or change elements within a list at any point in your program. This flexibility allows for dynamic data manipulation.
Recommendations for Effective List Usage
- List Functions: Python provides a variety of built-in functions for lists, such as append, remove, pop, sort, clear, etc. Understanding and utilizing these functions can streamline your list operations.
- For Loops with Lists: Using a for loop to iterate over a list can simplify displaying or processing all elements within the list efficiently.
Expert Advice for List Manipulation
- List Methods: Experiment with different list methods to gain a deeper understanding of how they work and how they can be applied in various scenarios.
- Practice Makes Perfect: The more you work with lists in Python, the more comfortable and proficient you will become in leveraging their power for data storage and manipulation.
Curiosity Question: How can you implement nested lists to create more complex data structures in Python?
By exploring these advanced insights and incorporating them into your Python programming practice, you can elevate your skills and proficiency in utilizing lists effectively for diverse tasks and projects.
Gaining mastery over lists in Python opens up a world of possibilities for data handling and manipulation. How will you leverage this knowledge to enhance your coding projects further?
Additional Resources for Python Lists
- Article: Python Lists Documentation
- Tutorial: W3Schools Python Lists Tutorial
- Video Tutorial: Python Lists Explained - YouTube Video
- Book: "Python Crash Course" by Eric Matthes (Chapter on Lists)
Dive deeper into the world of Python lists with these valuable resources. Explore different perspectives, tutorials, and examples to enhance your understanding and proficiency in working with lists in Python. Happy learning! 🐍📚
Practice
Task
task: Write a function called add_food_item
that takes a list of food items and a food item as arguments. The function should add the food item to the list and return the updated list.
task: Define a function remove_food_item
that takes a list of food items and a food item as arguments. The function should remove the food item from the list if it exists, and return the updated list. If the item is not found, return a message saying "Item not found".
task: Write a function sort_food_items
that takes a list of food items and sorts them in alphabetical order. The function should return the sorted list.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
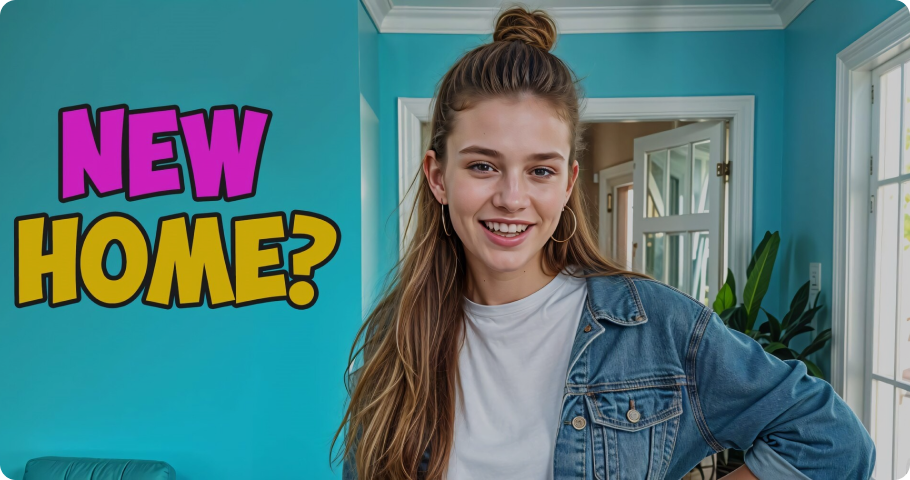
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.