Error Handling
Understand the concept of exceptions and how to handle them.
Introduction to Error Handling in Python
In this course, we will dive into error handling in Python, focusing on the concept of exceptions and how to handle them effectively.
When writing Python programs, you may encounter situations where something goes wrong, such as trying to open a file that doesn’t exist, dividing by zero, or accessing an index that’s out of range. These situations are called exceptions, and Python provides tools to handle them gracefully.
In this course, we will cover the following key topics:
- What exceptions are and why they occur
- The
try
,except
,else
, andfinally
blocks for handling exceptions - Raising exceptions intentionally with the
raise
keyword - Best practices for writing robust error-handling code
By the end of this course, you’ll be able to handle errors in your programs in a way that prevents crashes and provides a better experience for users. Let’s get started!
Main Concepts of Error Handling in Python
-
Exceptions: An exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. For example, dividing by zero or attempting to open a non-existent file can cause exceptions.
-
try and except: The
try
block allows you to test a block of code for errors, while theexcept
block lets you handle those errors.- Example:
In this example, the program will print 'Cannot divide by zero!' without crashing.try: x = 1 / 0 except ZeroDivisionError: print('Cannot divide by zero!')
- Example:
-
else: The
else
block, when used withtry
andexcept
, runs if no exception is raised in thetry
block.- Example:
try: x = 5 / 2 except ZeroDivisionError: print('Cannot divide by zero!') else: print('Division was successful!')
- Example:
-
finally: The
finally
block runs code that must be executed whether an exception occurs or not. It is often used for cleanup actions like closing files or releasing resources.- Example:
try: file = open('file.txt', 'r') except FileNotFoundError: print('File not found!') finally: print('This will always run.')
- Example:
-
Raising Exceptions: You can raise exceptions intentionally using the
raise
keyword. This is useful when you want to enforce specific conditions in your code.- Example:
def check_age(age): if age < 18: raise ValueError('Age must be 18 or older') return age
- Example:
By understanding and implementing these error-handling concepts, you can make your Python programs more reliable and user-friendly.
Practical Applications of Error Handling in Python
Handling Division by Zero
When performing division in Python, you might encounter a ZeroDivisionError
if you try to divide by zero. Here’s how you can handle it using try
and except
blocks:
try: result = 10 / 0 except ZeroDivisionError: print('Cannot divide by zero!') ``` This prevents your program from crashing and gives a helpful message instead. ### File Handling with Exception Handling When opening a file, you might encounter a `FileNotFoundError` if the file doesn’t exist. Here's an example: ```python try: file = open('nonexistent_file.txt', 'r') except FileNotFoundError: print('File not found!') finally: print('File handling complete.') ``` The `finally` block ensures that cleanup code (like closing the file) runs whether an error occurs or not. ### Raising Custom Exceptions You can also create your own exceptions to enforce certain conditions in your program. For example, you can raise a `ValueError` if a user inputs an invalid age: ```python def check_age(age): if age < 18: raise ValueError('Age must be 18 or older') return age ``` This ensures that only valid data is processed in your program. Experiment with these examples in your Python environment to see how error handling can make your code more robust and resilient.
Test your Knowledge
What does the try
block in Python do?
What does the try
block in Python do?
Advanced Insights into Error Handling in Python
Now that we've covered the basics of error handling, let's look at some advanced insights.
Creating Custom Exceptions
In Python, you can define your own exceptions by creating a custom class that inherits from the Exception
class. For example:
class NegativeNumberError(Exception): pass ``` You can then raise this exception whenever a negative number is encountered in your program. ### Exception Chaining Python supports exception chaining, where one exception can cause another. This is useful when you need to raise a new exception while preserving the original one. For example: ```python try: # some code except FileNotFoundError as e: raise CustomException('Custom error message') from e ``` This helps in debugging and understanding the flow of exceptions. ### Best Practices 1. **Catch Specific Exceptions**: Always catch the specific exception rather than using a generic `except:` block. This prevents masking other errors and ensures you're handling only the expected exceptions. 2. **Clean Up with `finally`**: Always use `finally` for cleanup operations like closing files or database connections. This ensures resources are released properly. #### Curiosity Question How would you handle a scenario where an exception occurs in a multi-threaded Python program? What strategies can you use to ensure thread safety while handling exceptions?
Additional Resources for Error Handling in Python
- Python's Official Documentation on Exceptions: Learn more about exceptions in Python from the official Python documentation.
- Python Try Except Tutorial: A hands-on guide for working with Python exceptions.
- Advanced Error Handling in Python: Learn how to create custom exceptions and handle more complex error scenarios.
- Python Error Handling Best Practices: Best practices for error handling in Python for robust and maintainable code.
Explore these resources to deepen your understanding of error handling in Python.
Practice
Task
task: Write a function that divides two numbers. Use error handling to manage division by zero.
task: Create a program that attempts to open a file. Use try
and except
blocks to handle the case where the file does not exist.
task: Write a function that raises a ValueError
if a number less than 10 is provided as an argument.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
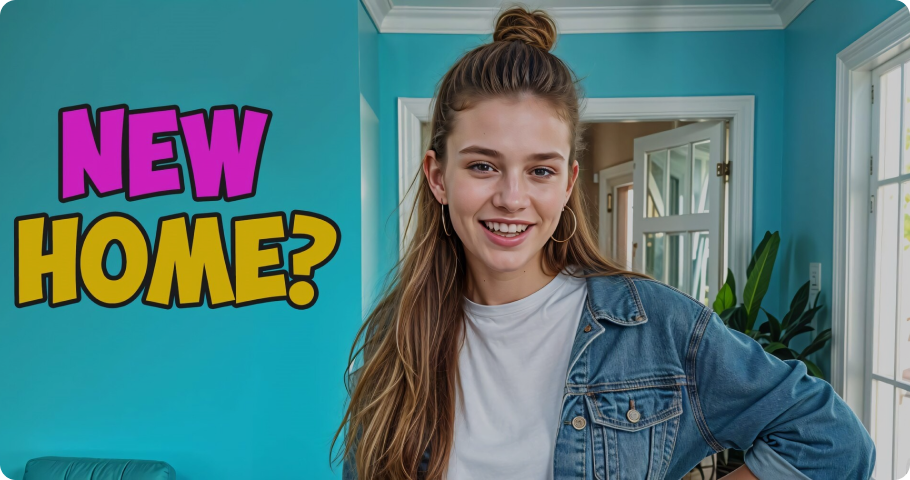
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.