Modules and Packages
In this module, we will explore how to create and use modules and packages in Python. A **module** is simply a Python file that contains functions, classes, and variables. Python provides a built-in mechanism to organize related code into these modules, making it easier to manage large programs. You'll also learn about **packages**, which are collections of related modules grouped together in a directory. By breaking your code into smaller, reusable pieces, you can improve code maintainability, readability, and scalability. We'll start by learning how to create custom modules, import functions from them, and organize your code into directories to create a package. Additionally, we'll look at how to use the special `__init__.py` file, which signals Python that a directory should be treated as a package. By the end of this module, you'll have the skills to structure your Python projects efficiently using modules and packages.
Lets Go!
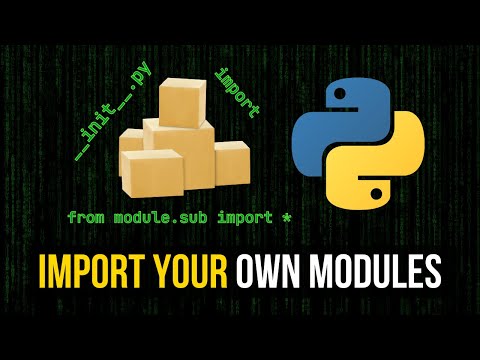
Modules and Packages
Level 7
In this module, we will explore how to create and use modules and packages in Python. A **module** is simply a Python file that contains functions, classes, and variables. Python provides a built-in mechanism to organize related code into these modules, making it easier to manage large programs. You'll also learn about **packages**, which are collections of related modules grouped together in a directory. By breaking your code into smaller, reusable pieces, you can improve code maintainability, readability, and scalability. We'll start by learning how to create custom modules, import functions from them, and organize your code into directories to create a package. Additionally, we'll look at how to use the special `__init__.py` file, which signals Python that a directory should be treated as a package. By the end of this module, you'll have the skills to structure your Python projects efficiently using modules and packages.
Get Started ๐Modules and Packages
In this module, we will explore how to create and use modules and packages in Python. A **module** is simply a Python file that contains functions, classes, and variables. Python provides a built-in mechanism to organize related code into these modules, making it easier to manage large programs. You'll also learn about **packages**, which are collections of related modules grouped together in a directory. By breaking your code into smaller, reusable pieces, you can improve code maintainability, readability, and scalability. We'll start by learning how to create custom modules, import functions from them, and organize your code into directories to create a package. Additionally, we'll look at how to use the special `__init__.py` file, which signals Python that a directory should be treated as a package. By the end of this module, you'll have the skills to structure your Python projects efficiently using modules and packages.
Introduction to Python Custom Modules
Welcome to this lesson on Python Modules and Packages! In this course, we'll learn how to break down your Python code into smaller, more manageable pieces using modules and packages. Organizing your code into these structures makes it easier to maintain and allows you to reuse functionality across multiple projects.
Modules in Python are simply files that contain Python code, and packages are directories that contain multiple related modules. By learning to structure your Python projects using these concepts, youโll be able to build scalable and efficient applications. Whether you're a beginner or looking to refine your knowledge, this course will help you harness the power of modular programming.
Have you ever found yourself repeating the same functions or pieces of code across multiple files? Wouldn't it be great if you could simply import them into other projects? Let's dive into Python modules and packages and see how we can organize our code to make it more efficient and reusable!
Main Concepts of Python Modules and Packages
-
Creating Custom Python Modules
- In Python, a module is simply a file containing Python code (functions, classes, or variables). You can create your own modules by saving your code in a
.py
file.
- In Python, a module is simply a file containing Python code (functions, classes, or variables). You can create your own modules by saving your code in a
-
Importing Functions from Modules
- You can import functions, variables, or entire modules into your Python script using the
import
statement. For example,import module_name
orfrom module_name import function_name
.
- You can import functions, variables, or entire modules into your Python script using the
-
Packages
- A package is a directory that contains multiple Python modules. A package allows you to organize related modules together. For Python to recognize a directory as a package, you must include an
__init__.py
file.
- A package is a directory that contains multiple Python modules. A package allows you to organize related modules together. For Python to recognize a directory as a package, you must include an
-
__init__.py
Files- The
__init__.py
file is essential for defining a directory as a package. It can be empty or contain initialization code, but its presence makes Python treat the directory as a package.
- The
-
Submodules
- You can organize modules within packages into submodules by creating subdirectories and adding additional
__init__.py
files to ensure everything works smoothly.
- You can organize modules within packages into submodules by creating subdirectories and adding additional
By understanding these concepts, you'll be able to structure your Python projects more effectively and easily reuse code across different parts of your application.
Practical Applications of Python Modules and Packages
Hereโs how you can create and organize Python modules and packages in your projects:
Step 1: Create Your Module
- Create a new Python file named
calculator.py
. - Define some basic arithmetic functions in it like
add()
,subtract()
,multiply()
, anddivide()
. - Save this file as
calculator.py
.
Step 2: Use Your Module in Another Script
-
In your main script (e.g.,
main.py
), import the functions fromcalculator.py
. -
Use these functions by calling them with their names like
add(5, 3)
.Example:
from calculator import add result = add(10, 20) print(result)
Step 3: Create a Package
- Create a directory called
math_package
. - Inside
math_package
, create two Python files:addition.py
andsubtraction.py
. - Add a function in each file for the respective operation.
- Add an empty
__init__.py
file to tell Python this directory is a package.
Step 4: Import from the Package
-
In your main script, import the
addition
andsubtraction
functions from the package. -
Use them in your code.
Example:
from math_package.addition import add from math_package.subtraction import subtract print(add(10, 5)) # 15 print(subtract(10, 5)) # 5
Step 5: Create Submodules within a Package
- You can further organize your code by adding subdirectories within
math_package
and creating more Python files. - These submodules can then be imported in the same way.
Experiment with creating your own custom modules and packages. Try organizing functions into different modules and use packages to structure your codebase more effectively!
Test your Knowledge
What is a Python module?
What is a Python module?
Advanced Insights into Python Modules and Packages
Understanding the underlying structure and organization of Python modules and packages is crucial for building scalable applications. Letโs dive into some advanced concepts:
-
Relative and Absolute Imports: When working with modules within packages, itโs important to understand the difference between absolute and relative imports. Absolute imports refer to the full path to the module, while relative imports use the location of the current module to reference other modules in the same package.
-
Namespace Packages: Python supports namespace packages, which allow you to organize multiple directories into a single logical package, even if those directories are located in different places on your filesystem.
-
Modular Codebase Design: As your project grows, organizing your code into well-defined modules and packages can make your project easier to manage, test, and extend. A modular codebase also allows for better collaboration since different team members can work on different parts of the code without interfering with each other.
By mastering these techniques, youโll be able to handle larger projects and improve your ability to structure and organize your code effectively.
Additional Resources for Python Modules and Packages
- Python Documentation on Modules - Official Python documentation explaining how modules work in Python.
- Real Python: Python Packages and Modules
- GeeksforGeeks: Python Modules - A comprehensive guide on Python modules, including how to create and use them effectively.
- Python Module of the Week - A collection of Python modules with examples and explanations for deeper understanding.
Practice
Task
Task: Write a Python script to create a module greetings.py
that defines a function say_hello()
. Import this module into another script and call the function to print a greeting.
Task: Create a package shapes
that includes modules for different shapes (e.g., circle.py
, square.py
). Each module should have a function that calculates the area of the respective shape. Import and use these functions in your main script.
Task: Create a submodule geometry
inside the package shapes
. Include functions like perimeter()
in geometry.py
. Import the function into your main script and use it.
Task: Write a Python program that defines a package math_operations
containing two submodules: addition.py
and multiplication.py
. Use these submodules in your main program to perform operations.
Task: Organize a set of utility functions (e.g., for file handling, data processing) into a package called utils
. Create several modules within utils
and import specific functions into your main script.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
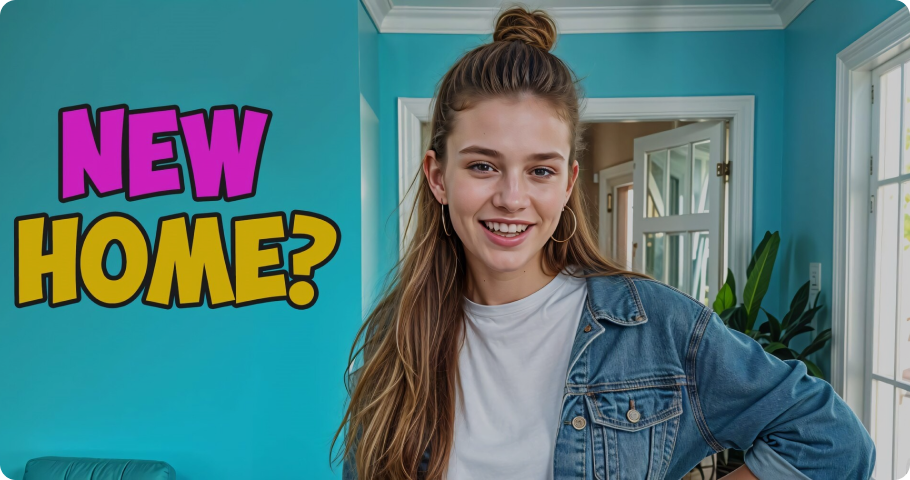
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.