Testing
Unit testing is a crucial aspect of software development that ensures the reliability and functionality of your code.
Lets Go!
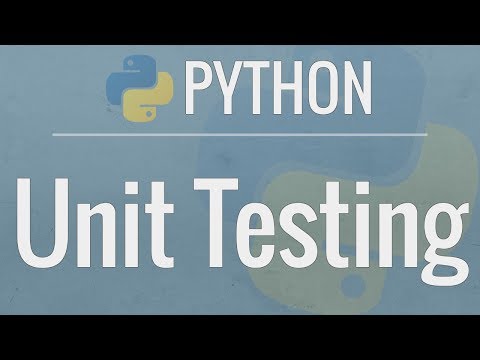
Testing
Level 9
Unit testing is a crucial aspect of software development that ensures the reliability and functionality of your code.
Get Started 🍁Testing
Unit testing is a crucial aspect of software development that ensures the reliability and functionality of your code.
Introduction to Unit Testing in Python
Welcome to the "Introduction to Unit Testing in Python" course!
Unit testing is a crucial aspect of software development that ensures the reliability and functionality of your code. In this course, we will delve into the fundamentals of unit testing in Python, covering topics such as writing tests, setting up and tearing down tests, and following best practices.
Have you ever wondered how to verify that your code works as expected without manual testing? Unit testing provides a systematic approach to validate individual units of code, ensuring that they perform as intended and remain error-free.
Throughout this course, you will learn the importance of testing your code, how to create robust and effective unit tests, and how to incorporate testing into your development workflow. By mastering unit testing, you can boost your confidence in the stability of your code and streamline the debugging process.
Whether you are a beginner looking to enhance your Python skills or an experienced developer seeking to level up your testing toolkit, this course will equip you with the knowledge and techniques needed to become proficient in unit testing.
Get ready to embark on a journey to harness the power of unit testing in Python and elevate your coding practices to new heights. Let's dive in and start testing your code with precision and confidence!
Main Concepts of Unit Testing in Python
- Definition of Testing: Testing code involves writing tests, setting up and tearing down tests, and following best practices to ensure code quality.
- Importance of Testing: Thoroughly testing code is essential to catch errors, ensure updates and refactoring don't break existing code, and save time in the long run.
- Unit Testing Module: Python comes with a built-in unit testing module that allows developers to write and run tests efficiently.
- Writing Tests: Tests can be written using the
unittest
module, with methods starting withtest_
and employingassert
statements to check for expected outcomes. - Automating Tests: By using proper test naming conventions, tests can be automated and conveniently run from the terminal or within the code editor.
- Setup and Teardown: Utilizing
setup
andteardown
methods helps to create and clean up test environments, ensuring test isolation and ease of maintenance. - Mocking: Mocking is a technique used to simulate external dependencies, like network calls, in order to isolate tests from external factors and focus on code behavior.
- Best Practices: It is important to follow best practices in unit testing, including test isolation, test-driven development, and continuous improvement of testing strategies.
Practical Applications of Unit Testing in Python
Unit testing in Python can save you time and prevent headaches by ensuring your code works as expected with each update or refactoring. Here's a step-by-step guide to get you started with writing tests using the built-in unit testing module.
Step 1: Write Simple Functions
Start by creating simple functions that you want to test. For example, you can create an add
function that adds two numbers together.
Step 2: Create a Test Module
- Create a new file in the current directory and name it
test_calc.py
. - Import the
unittest
module and the module you want to test (e.g.,calc
) in your test module.
Step 3: Write Test Cases
- Create a test class that inherits from
unittest.TestCase
(e.g.,TestCalc
). - Write test methods starting with
test_
to test your functions (e.g.,test_add
). - Use
self.assertEqual()
to compare the actual output of your function with the expected output.
Step 4: Run Your Tests
- Run your tests from the command line using
python -m unittest test_calc
. Make sure all tests pass. - To run tests within your editor, add the following code at the bottom of your test module:
if __name__ == '__main__': unittest.main()
Step 5: Use Setup and Teardown
- Use
setUp()
andtearDown()
methods to set up and clean up test environments before and after each test. - Reuse common setup steps for multiple tests to keep your code DRY.
Step 6: Implement Mocking
- Use
unittest.mock.patch
to mock external dependencies such as web requests. - Mock external calls to simulate both successful and failed responses for thorough testing.
Step 7: Practice Isolation and Test-Driven Development
- Ensure that each test is isolated and independent of others to avoid interference.
- Experiment with Test-Driven Development by writing tests before implementing code to guide development.
By following these steps, you can enhance the quality of your code, catch bugs early, and build robust applications in Python. Give it a try and see the benefits of incorporating unit testing into your development workflow!
Test your Knowledge
What is the primary purpose of unit testing?
What is the primary purpose of unit testing?
Advanced Insights into Unit Testing
Unit testing in Python can be taken to a more advanced level by incorporating concepts like setup and teardown methods, mocking, and test-driven development. Here are some key insights to enhance your unit testing skills:
Setup and Teardown Methods
- Setup Method: The
setUp
method runs before every test, allowing you to set up any necessary resources or objects. - Teardown Method: The
tearDown
method runs after every test, enabling you to clean up resources or perform any necessary post-test actions.
Mocking
- Purpose: Mocking allows you to simulate external dependencies, such as web requests, to control the behavior of your code during testing.
- Usage: Patching objects with the
patch
method helps in replacing functionalities like making requests to a website with predefined responses, ensuring your tests are not affected by external factors.
Test-Driven Development
- Concept: Test-Driven Development (TDD) involves writing tests before writing the actual code, ensuring that the code is built to meet specific requirements set by the tests.
- Benefit: TDD helps in maintaining code quality, ensuring code coverage, and validating the functionality against expected outcomes.
Best Practices
- Isolated Tests: Tests should be independent of each other to avoid dependencies and ensure that each test can run successfully on its own.
- Incremental Testing: Start with basic assertions and gradually move towards more complex scenarios, incorporating setup and teardown methods as needed.
By incorporating these advanced insights into your unit testing practices, you can enhance the robustness and reliability of your code tests, leading to improved code quality and faster debugging processes.
Additional Resources for Unit Testing in Python
-
Python Unit Testing Documentation - The official Python documentation on unit testing provides in-depth information on the built-in unit testing module.
-
Guide to Writing Good Unit Tests in Python - This article on Real Python offers tips and best practices for writing effective unit tests in Python.
-
Mocking in Python with unittest.mock - Learn more about mocking in Python using the
unittest.mock
module in the official Python documentation. -
Introduction to Test-Driven Development (TDD) - Understand the concept of Test-Driven Development (TDD) and the benefits of writing tests before writing code.
-
Comparing unittest and pytest in Python - Explore the differences between the
unittest
module and thepytest
framework in Python through this comprehensive article on Real Python.
Feel free to explore these resources to enhance your understanding of unit testing in Python and improve your testing practices. Happy testing!
Practice
Task
Task: Write a Python function that adds two numbers together. Create a unit test to ensure the function works as expected.
Task: Create a function that checks if a number is even. Write a unit test to confirm the result for multiple inputs.
Task: Implement a function that divides two numbers and returns the result. Write tests for valid division and division by zero.
Task: Create a program to test a simple function that multiplies two numbers. Use assertEqual()
to check for expected output.
Need help? Visit https://aiforhomework.com/ for assistance.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master Java Programming
Discover the power of Java to build cross-platform applications, develop robust software, and design scalable systems with ease.
Master Ruby Programming
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Master the Art of Go Programming
Dive into Golang and learn how to create fast, efficient, and scalable applications. Master the simplicity of Go for building backend systems, networking tools, and concurrent applications.
Master the Art of Figma Design
Dive into Figma and learn how to design beautiful, user-friendly interfaces with ease. Master Figma's powerful tools for creating high-fidelity prototypes, vector designs, and collaborative design workflows.
Completed the introduction to design Principles?
Here's what's up next! Continue to accelerate your learning speed!
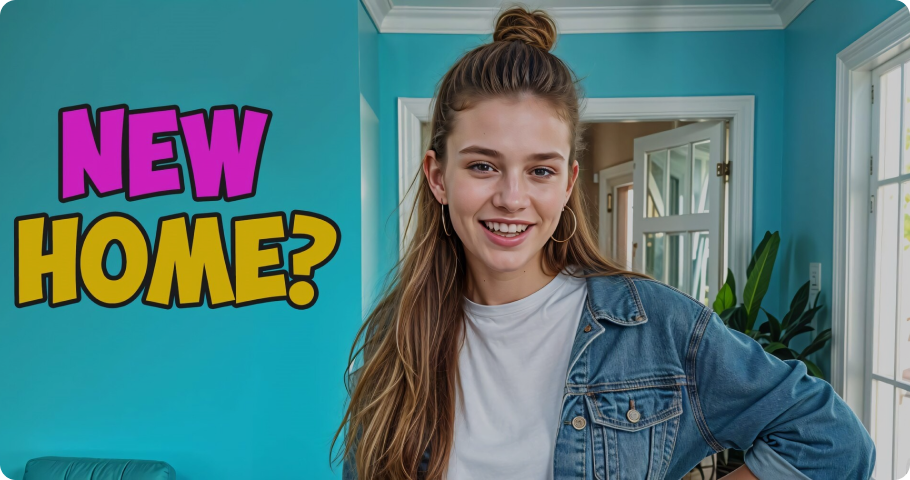
Wireframing Basics For UI/UX
Learn the foundational skills of wireframing to create clear, effective design layouts. This course covers essential techniques for sketching user interfaces, planning user flows, and building a solid design foundation.