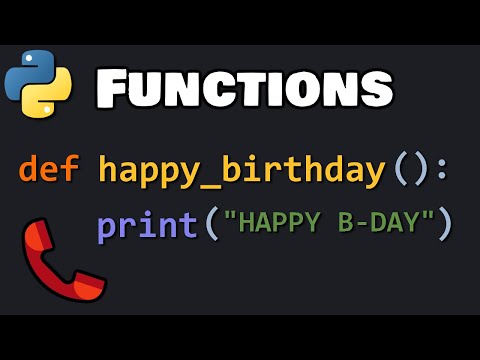
Defining and calling functions
Lesson 3
Understand how to define and call functions in Python.
Get Started 🍁Introduction to Functions in Python
Welcome to the course on Introduction to Functions in Python!
In this course, we will explore the fundamental concept of functions in Python.
Functions can be thought of as blocks of reusable code that can be invoked by placing a set of parentheses after the function name. They allow us to streamline our code and make it more efficient by avoiding repetition and enhancing modularity.
Have you ever found yourself needing to perform a specific task multiple times within your code? Functions are the perfect solution to such scenarios. Just imagine being able to write a piece of code once and then reuse it whenever you need to without duplicating it or resorting to cumbersome loops.
Throughout this course, we will delve into various aspects of functions, including defining functions, passing arguments, setting up parameters, and utilizing the return
statement to send data back to the caller.
Are you ready to enhance your Python programming skills and discover the power of functions? Let's embark on this learning journey together! 🚀
But first, let's address the question: How can functions simplify and optimize your coding experience? Join us as we unravel the magic of functions in Python!
Main Concepts of Functions in Python
-
Functions as Reusable Code: Functions in Python are blocks of code that can be reused by calling them whenever needed. This helps in avoiding repetitive code and improves the organization of your program.
-
Defining a Function: To define a function, you use the
def
keyword followed by a unique function name, parentheses, and a colon. Any code that belongs to the function should be indented underneath. -
Invoking a Function: To invoke a function, you simply type the function name followed by a set of parentheses. This tells Python to execute the code inside the function.
-
Arguments and Parameters: You can send data directly to a function using arguments. These are values or variables that you pass within the parentheses when calling a function. Parameters are placeholders inside the function that receive these arguments.
-
Returning a Value: The
return
statement is used to end a function and send a result back to the caller. This allows you to capture the output of a function and use it in other parts of your program. -
Order Matters: The order of arguments and parameters when invoking a function is crucial. They need to match in order for the function to work correctly.
-
Example - Creating an Invoice: An example is given where a function to display an invoice is created with three parameters: username, amount, and due date. This demonstrates how arguments are passed and used within a function.
-
Creating Complex Functions: Functions can perform complex operations, such as adding, subtracting, multiplying, or dividing numbers. You can define functions to perform specific tasks and return the results for further use.
-
Example - Creating a Full Name: A function is created to generate a full name by capitalizing the first and last names passed as arguments. This showcases how functions can manipulate data and return a formatted result.
Practical Applications of Functions
Functions in Python serve as reusable blocks of code that can be invoked with specific inputs, known as arguments. These arguments are matched with parameters defined in the function to perform tasks efficiently. Here are some practical applications of functions:
Step-by-Step Guide:
- Create a Function:
- Define a function using
def
followed by a unique function name and parentheses. - Indent the code that belongs to the function under the function definition.
- Define a function using
def happy_birthday(name, age): message = f"Happy Birthday to {name}. You are {age} years old!" return message
- Invoke the Function:
- Call the function by typing the function name, followed by parentheses containing the arguments.
- Ensure that the arguments match the parameters in the function definition.
print(happy_birthday("Bro", 20)) print(happy_birthday("Steve", 30)) print(happy_birthday("Joe", 40))
- Understand Return Statements:
- Use the
return
statement to send a result back from the function to the caller. - This allows you to capture and utilize the output of the function in your code.
- Use the
def add_numbers(x, y): result = x + y return result
- Create More Complex Functions:
- Define functions that perform more intricate tasks, such as capitalizing names or calculating values.
def create_full_name(first, last): first = first.capitalize() last = last.capitalize() full_name = f"{first} {last}" return full_name
- Put It All Together:
- Utilize functions in your Python code to streamline repetitive tasks and improve code organization.
- Experiment with different inputs and outputs to see the versatility of functions.
print(create_full_name("john", "doe")) print(create_full_name("spongebob", "squarepants"))
Ready to Try It Out?
Go ahead and copy the code snippets provided, paste them into your Python environment, and run them to see functions in action! Modify the inputs and explore how functions can enhance the efficiency and readability of your code. Happy coding! 🐍🚀
Test your Knowledge
What is the purpose of the def
keyword in Python?
What is the purpose of the def
keyword in Python?
Advanced Insights into Functions
Now that we have a solid understanding of functions, let's delve into some more advanced aspects that can enhance our coding skills.
Handling Multiple Arguments
When passing data to a function as arguments, it's essential to ensure that the number of arguments matches the number of parameters defined in the function. The order in which arguments are passed also matters, so make sure to maintain consistency to avoid errors.
Tip: Naming parameters descriptively can make your code more readable and help you understand the function's purpose at a glance.
Curiosity Question: How can you handle functions with a variable number of arguments, known as variable-length argument lists, to increase flexibility in your code?
Utilizing the Return Statement
The return statement not only ends the function but also allows you to send data back to the caller. By returning a value, you can perform calculations, transformations, or any task within a function and pass that result back to the main program.
Recommendation: Experiment with different types of calculations within functions and utilize the return statement to gain a better understanding of how to structure your code effectively.
Curiosity Question: In what scenarios would you prioritize using functions with return statements over functions that simply perform actions without returning any values?
Enhancing Readability with Parameter Names
Choosing intuitive and descriptive names for your parameters can significantly improve the readability and maintainability of your code. Clear parameter names make it easier for you and other developers to understand the purpose of each function and its expected inputs.
Expert Advice: Aim for clarity and precision in naming your parameters to enhance the overall quality of your code and facilitate collaboration with team members.
Curiosity Question: How can you apply meaningful parameter names that not only describe the data being passed but also convey the intended functionality of the function itself?
By exploring these advanced insights into functions, you can elevate your programming skills and optimize the efficiency of your code while maintaining clarity and organization. Practice incorporating these concepts into your Python projects to reinforce your understanding and become more proficient in utilizing functions effectively.
##Additional Resources for Functions in Python
- Article: Python Functions
- Video Tutorial: Functions in Python - Tutorial
- Documentation: Python Function Documentation
- Practice Exercises: Python Functions - Exercises
Expand your knowledge of functions in Python by exploring these additional resources. Whether you prefer articles, video tutorials, official documentation, or hands-on practice exercises, these resources will help you enhance your understanding of this fundamental concept in Python programming. Dive deeper into functions and unlock the full potential of your coding skills!
Practice
Task
Task: Write a function that takes two numbers as arguments and returns their sum.
Task: Create a function that takes a first name and last name and returns a formatted full name.
Task: Define a function that checks if a number is even or odd and returns an appropriate message.
Looking to master specific skills?
Looking for a deep dive into specific design challenges? Try these targeted courses!
Learn Ruby Programming Online with Step-by-Step Video Tutorials
Discover the elegance of Ruby to build dynamic web applications, automate tasks, and develop scalable solutions with simplicity and ease.
Learn Java Programming Online with Step-by-Step Video Tutorials
Explore our Java Programming course online with engaging video tutorials and practical guides to boost your skills and knowledge.
JavaScript Programming for Web Development
Master JavaScript to build dynamic, responsive websites and interactive web applications with seamless user experiences.
Master C ++ Programming
Unlock the power of C++ to create high-performance applications, develop advanced software, and build scalable systems with precision and control.
Master PHP Programming
Unlock the full potential of PHP to build dynamic websites, develop powerful web applications, and master server-side scripting.
Python Programming
Learn how to use Python for general programming, automation, and problem-solving.